By: CS2103T-W12-02
Since: Jan 2020
Licence: MIT
- 1. Introduction
- 2. About
- 3. How To Use
- 4. Overview of Features
- 5. Setting up
- 6. Design
- 7. Implementation
- 8. Documentation
- 9. Testing
- 10. Dev Ops
- Appendix A: Product Scope
- Appendix B: User Stories
- Appendix C: Use Cases
- Appendix D: Non Functional Requirements
- Appendix E: Glossary
- Appendix F: Product Survey
- Appendix G: Instructions for Manual Testing
- Appendix H: Effort
1. Introduction
Welcome to TeaPet!
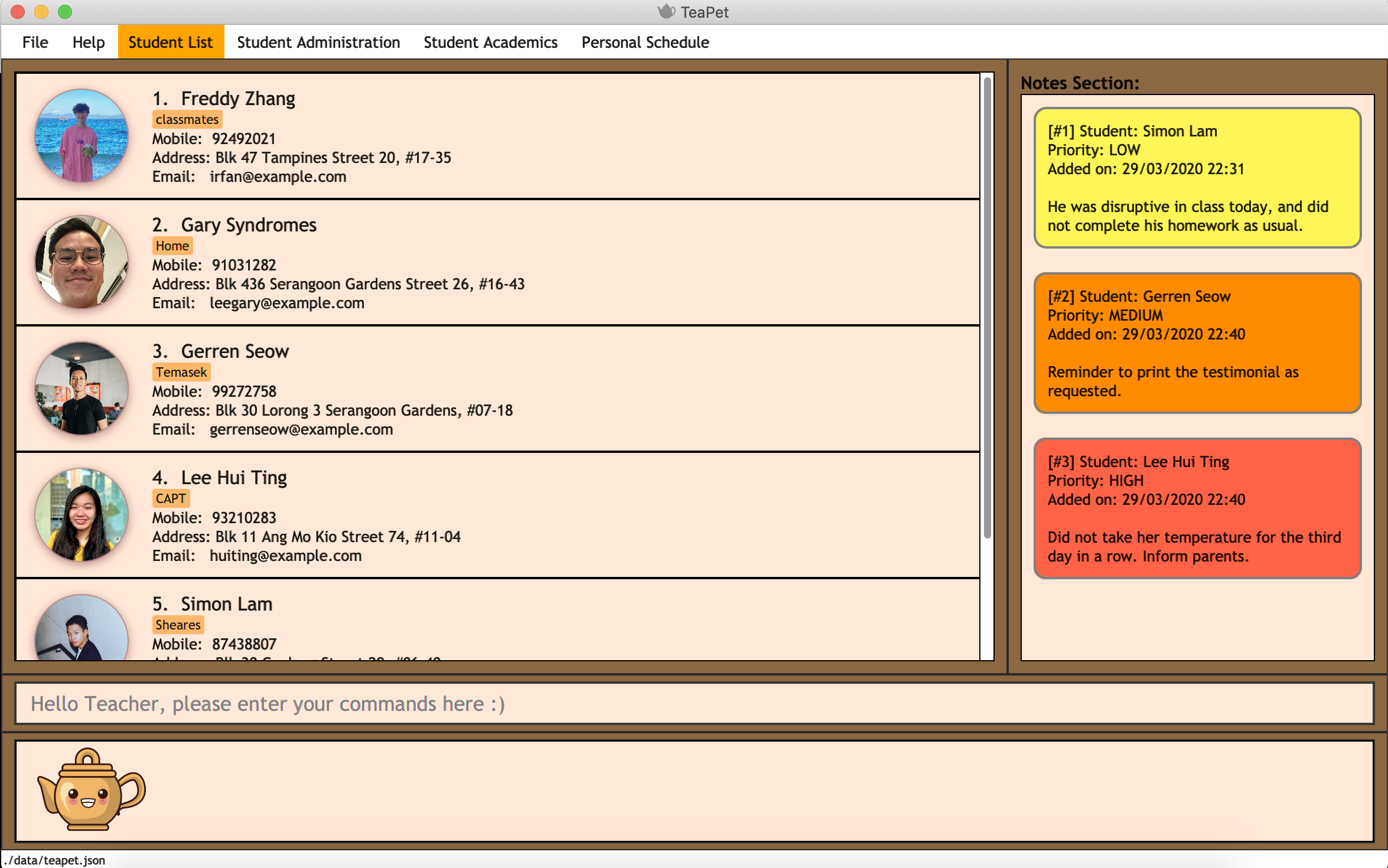
TeaPet is an integrated platform fully customized for Form Teachers in Primary, Secondary and Tertiary institutions to help them manage their classroom effectively. It contains their personal curriculum schedule, students' particulars, and can also track their class' academic progress.
All their important information is comfortably compartmentalized on our simple and clean Graphical User Interface (GUI) and we are optimized for users who are very comfortable working on the Command Line Interface (CLI).
If you are looking for a way to easily manage your administrative classroom chores and have quick hands, then TeaPet is definitely for you!
2. About
The aim of this Developer Guide is to provide an introduction to the software architecture and implementation of TeaPet. It is made for developers who wish to understand and maintain the backbone of our application.
At TeaPet, we prioritise teamwork. We welcome you developers to contribute and improve on our application anytime by opening an issue should you have any refreshing ideas to improve TeaPet after reading this guide.
3. How To Use
This section will teach you how should you go about understanding our Developer Guide in order to best understand our product.
Reading ahead, you will find that we’ve adopted the "top-down" approach into the structure of our Developer Guide — We will first look at the high-level architecture of our application before delving into the implementation details of each feature that makes up TeaPet.
We encourage you to read every page diligently from the top to the bottom in order to get the highest level of understanding of our application. If you are only interested in how we develop one feature, you may read the high-level design then focus on the implementation of that particular feature.
Take note of the following symbols and formatting used in this document:
This is used to show warnings |
This is used to show descriptions that the user should take note of. |
This symbol indicates tips. |
Enter |
This symbol indicates the Enter button on your keyboard. |
|
A grey highlight indicates that this is a command that can be typed into the command line and executed by the program. |
4. Overview of Features
This section will provide you a brief overview of TeaPet’s cool features and functionalities.
-
Manage your students easily
-
Include student’s particulars. e.g. address, contact number, next of kin (NOK).
-
Include administrative details of the students. e.g. attendance, temperature.
-
-
Plan your schedule easily
-
Create and manage your events with a single calendar.
-
View calendar at a glance.
-
-
Manage your class academic progress easily
-
Include every student’s grades for every examination.
-
Easy to track progress using helpful tools. e.g. graph plots.
-
-
Add Notes to act as lightweight, digital reminders easily
-
Include reminders for yourself to help you remember important information.
-
Search keywords in your notes.
-
Save the notes as administrative or behavioural.
-
-
Data is saved onto your hard disk automatically
-
Any changes made will be saved onto your computer so you dont have to worry about data being lost.
-
5. Setting up
This section provides you with the tools needed for you to set up TeaPet.
You can refer to the guide here.
6. Design
6.1. Architecture
This section describes the high-level software architecture of TeaPet.
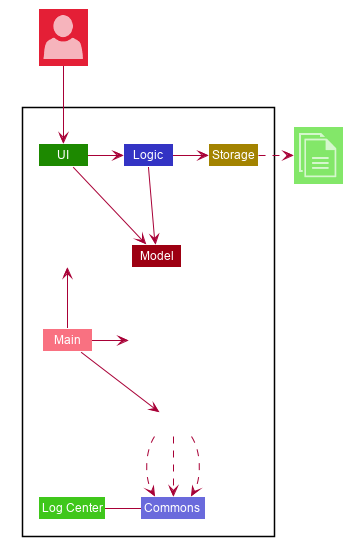
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.
The .puml files used to create diagrams in this document can be found in the diagrams folder.
Refer to the Using PlantUML guide to learn how to create and edit diagrams.
|
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components.
The following class plays an important role at the architecture level:
-
LogsCenter
: Used by many classes to write log messages to the App’s log file.
The rest of the App consists of four components.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name} Manager
class.
For example, the Logic
component (see the class diagram given below) defines it’s API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
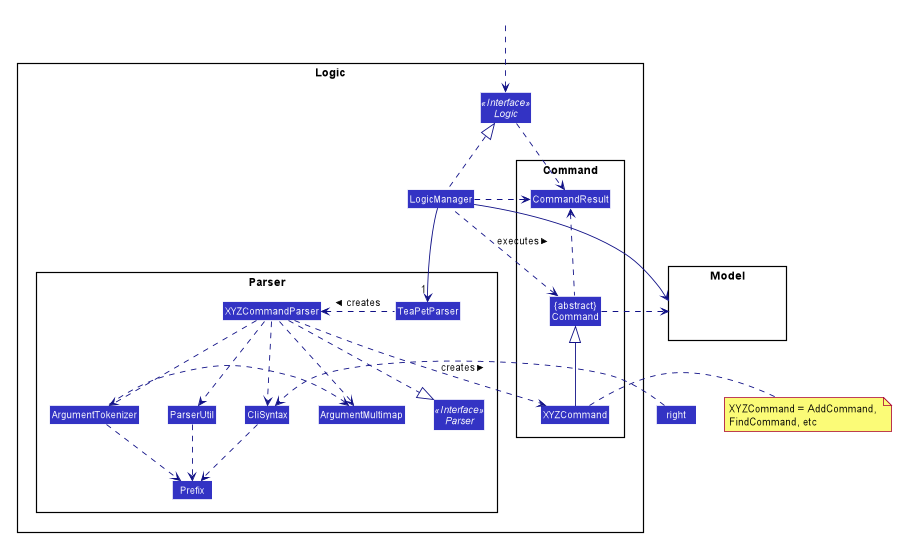
How the architecture components interact with each other
The Sequence Diagram below shows how the components interact with each other for the scenario where the user issues the command {Entity Name} student delete 1
.
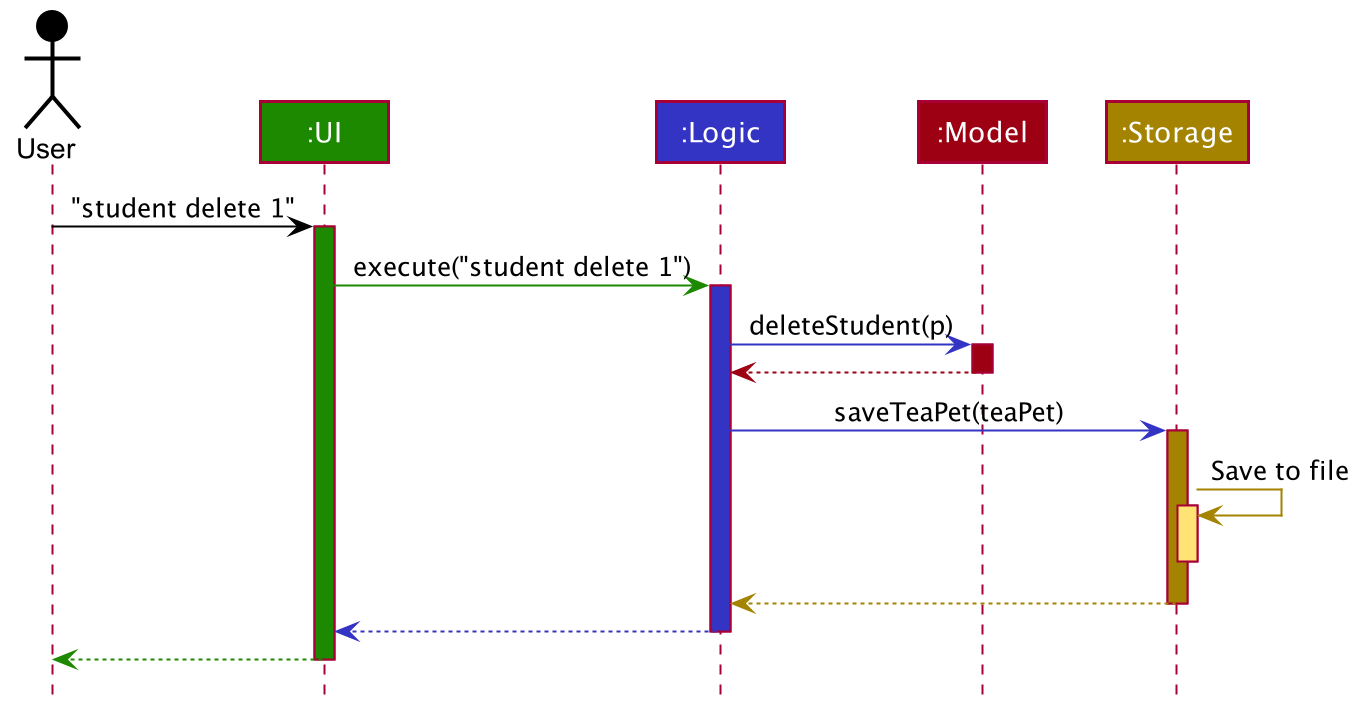
student delete 1
commandThe sections below give more details of each component.
6.2. UI component
This section describes the high-level software structure of TeaPet’s UI Component.
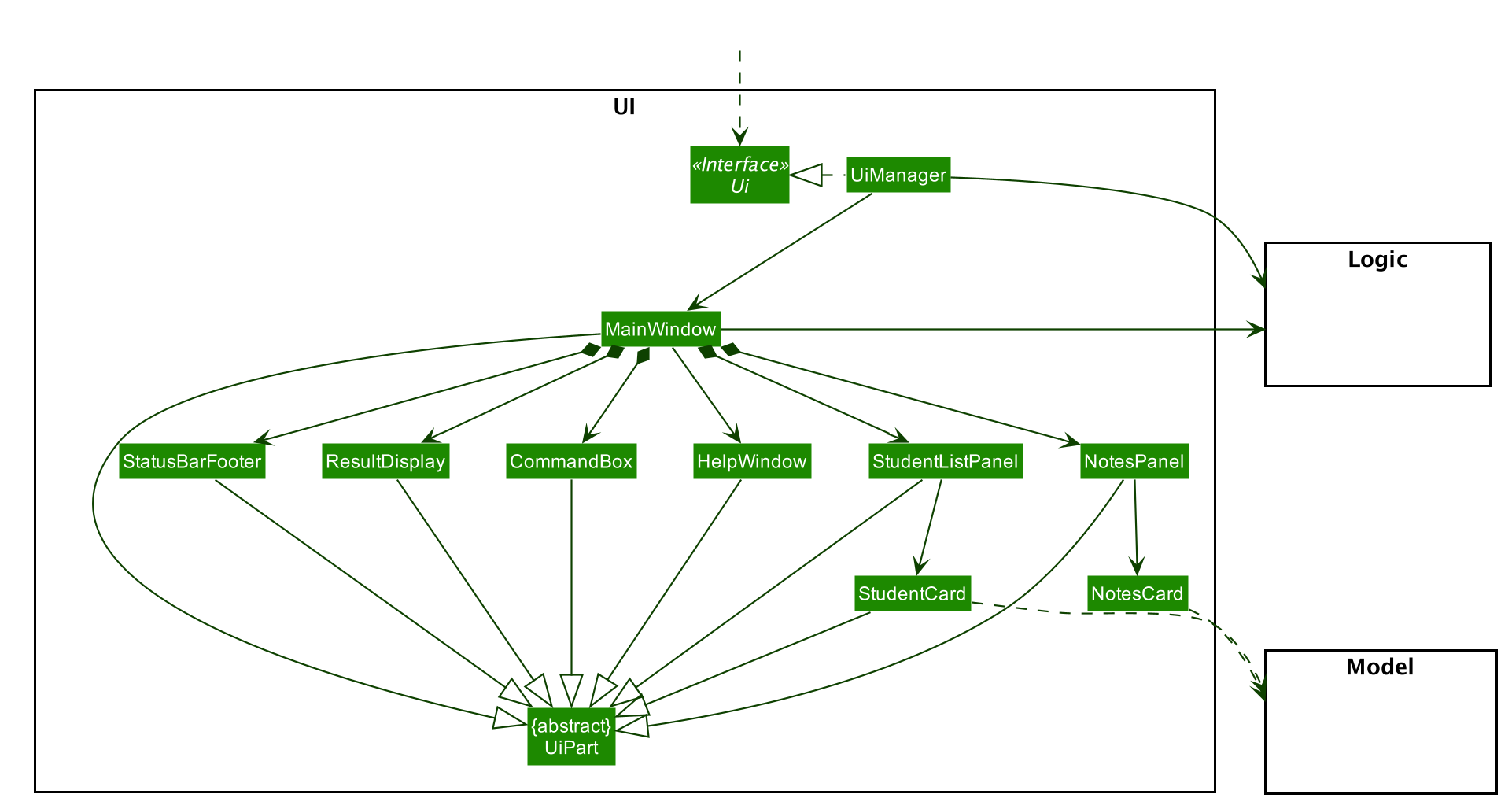
API : Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, StudentListPanel
, NotesPanel
, StatusBarFooter
and HelpWindow
. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
-
Executes user commands using the
Logic
component. -
Listens for changes to
Model
data so that the UI can be updated with the modified data.
6.3. Logic component
This section describes the high-level software structure of TeaPet’s Logic Component.
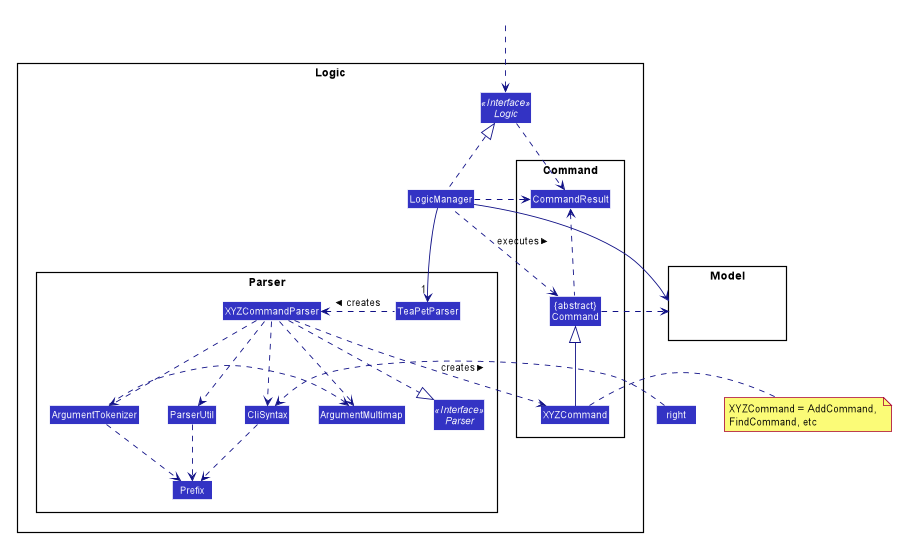
API :
Logic.java
-
Logic
uses theTeaPetParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a student). -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUI
,and then displayed to the user. -
In addition, the
CommandResult
object can also instruct theUI
to perform certain actions.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("student delete 1")
API call.
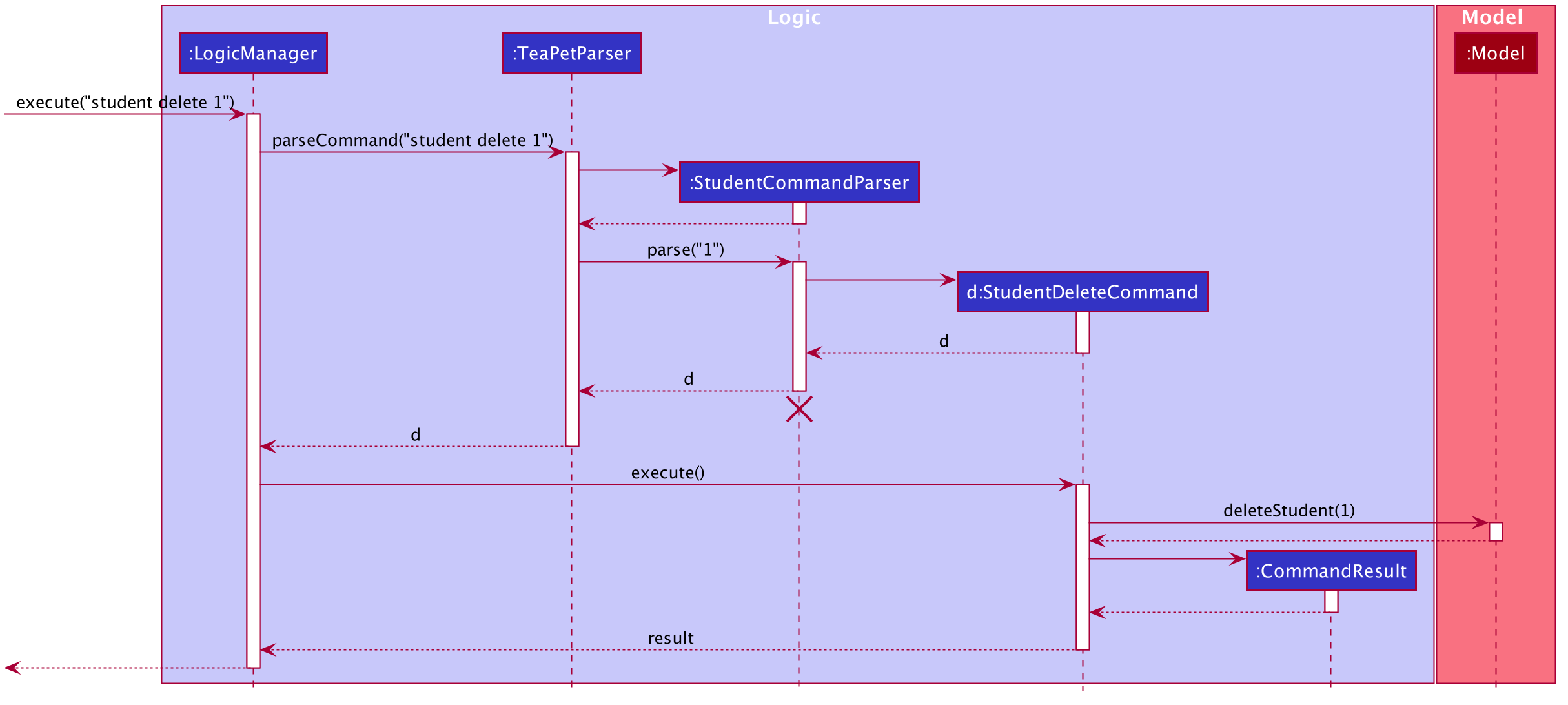
student delete 1
Command
The lifeline for StudentCommandParser should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
|
6.4. Model component
This section describes the high-level software structure of TeaPet’s Model Component.
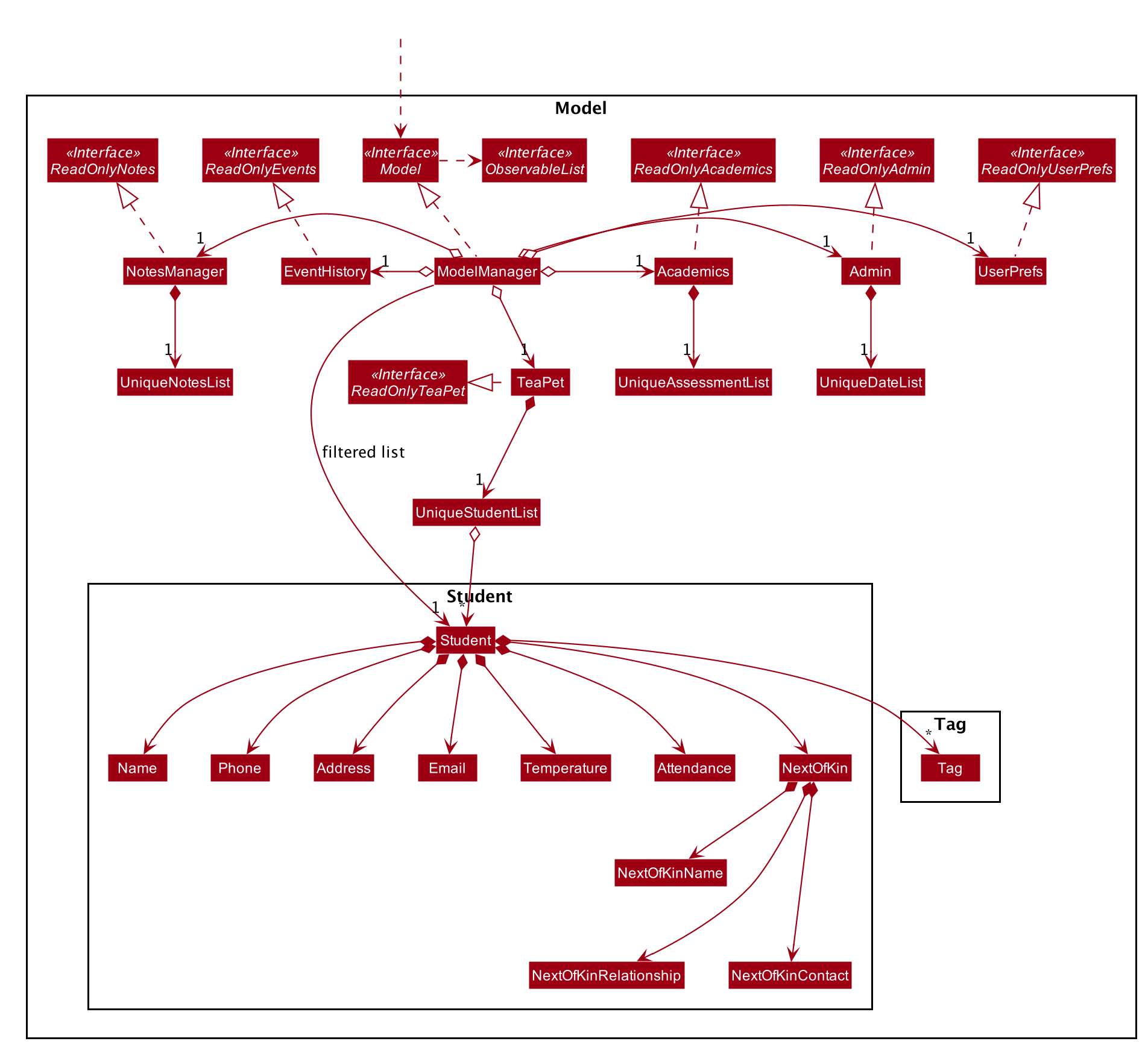
Student
class as a detailed example.API : Model.java
The Model
,
-
stores a
UserPref
object that represents the user’s preferences. -
stores the data of different Entities.
-
stores in-memory data of Students, Admin, Academics, Notes and Events.
-
exposes multiple unmodifiable
ObservableLists
that can be 'observed' e.g. the UI can be bound to these lists so that the UI automatically updates when the data in the lists change. -
does not depend on any of the other three components.
6.5. Storage component
This section describes the high-level software structure of TeaPet’s Storage Component.
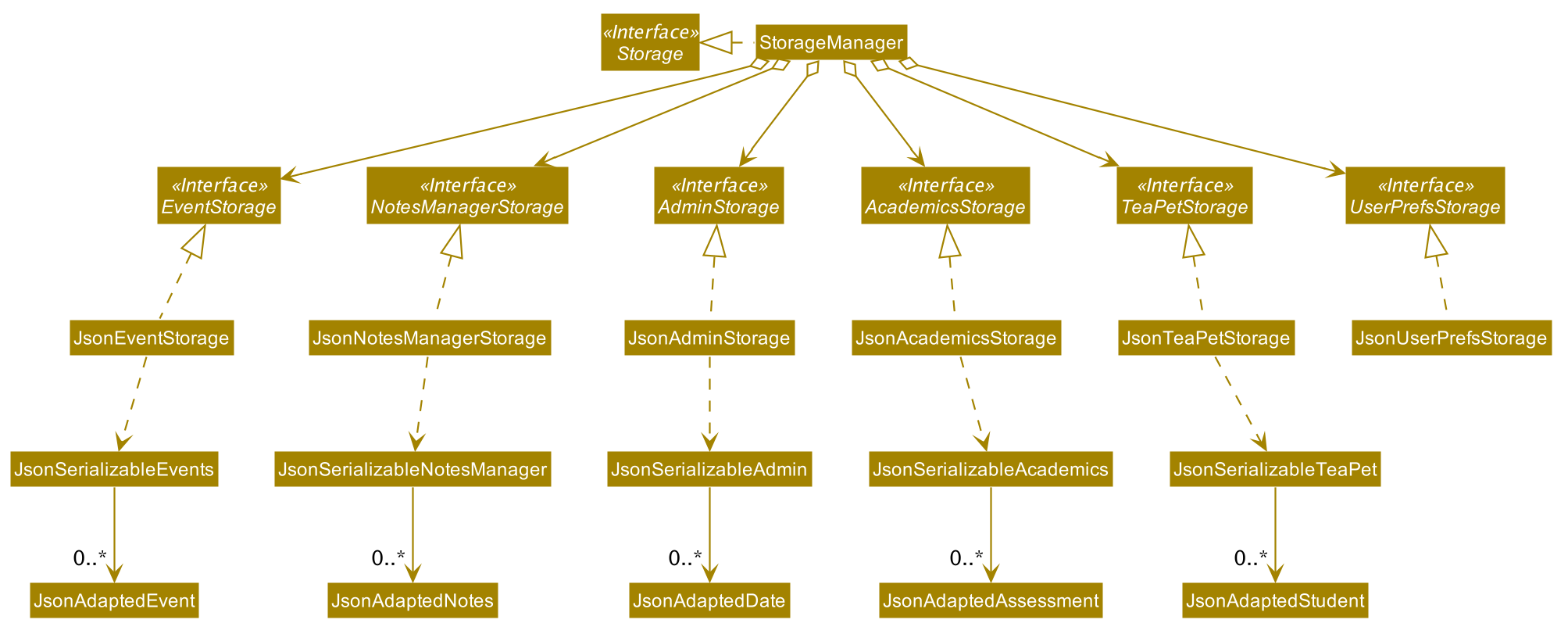
API : Storage.java
The Storage
component,
-
converts Model objects into savable data in JSON-format and vice versa.
-
can save
UserPref
objects in json format and read it back. -
can store
Students
,Admin
,Academics
,Notes
andEvents
in a several json files, which can be read.
6.6. Common classes
Classes used by multiple components are in the seedu.address.commons
package.
7. Implementation
This section describes some noteworthy details on how certain features are implemented.
7.1. Student particulars feature
The student particulars feature keeps track of essential student details. The feature comprises of commands namely,
-
StudentAddCommand
- Adds the student particulars into the class list -
StudentEditCommand
- Edits the particulars of a student -
StudentDeleteCommand
- Deletes the student information -
StudentFindCommand
- Finds information of the required student -
StudentClearCommand
- Deletes all student details from the student list
The student commands all share similar paths of execution and is illustrated in the following sequence diagram below, which shows the sequence diagram for the StudentAddCommand.
The commands when executed, will interface with the methods exposed by the Model
interface to perform the related operations
(See logic component for the general overview).
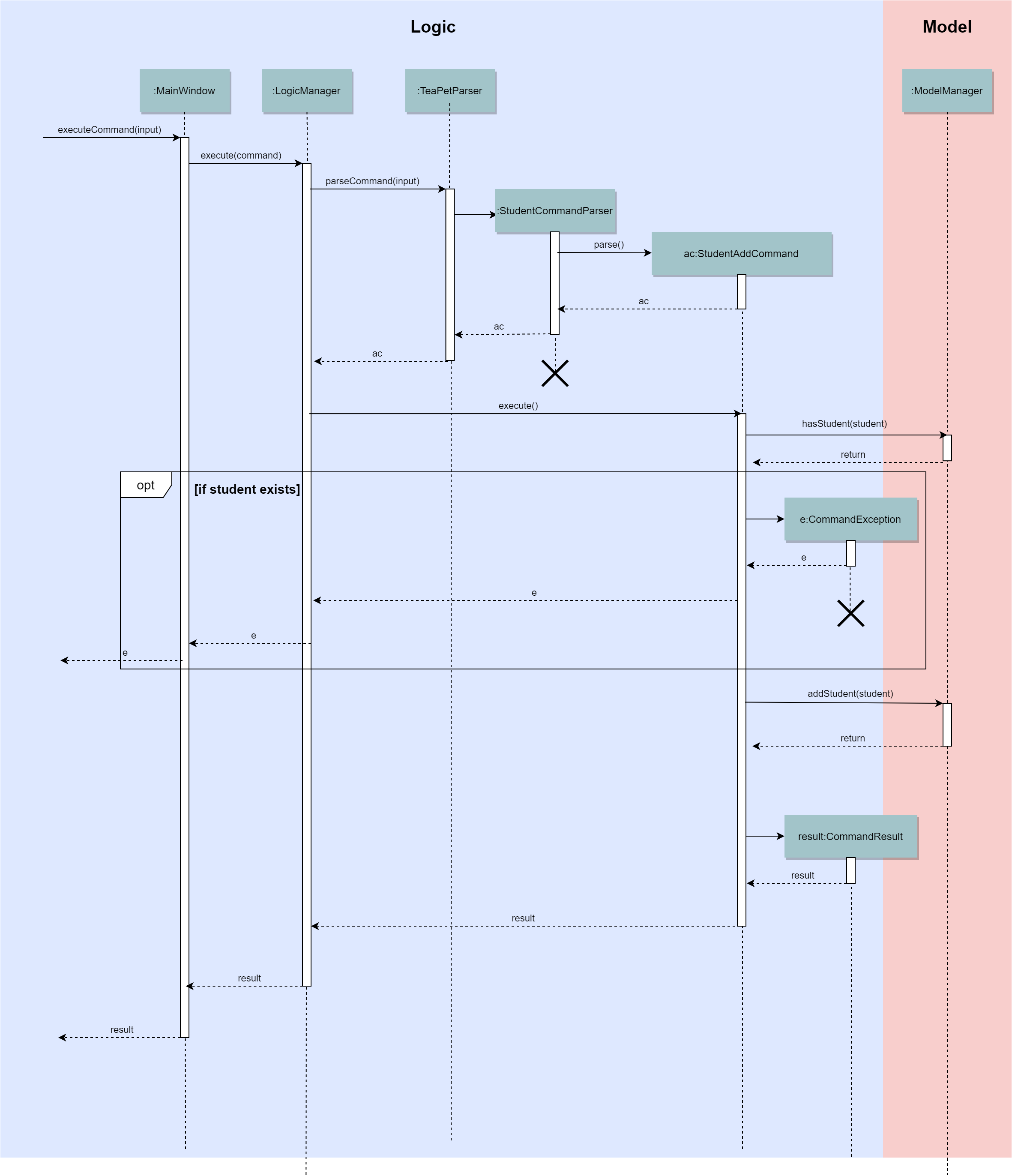
These are the common steps among the Student Commands:
-
The
TeaPetParser
will assign theStudentCommandParser
to parse the user input. -
The
StudentCommandParser#parse
will take in a string of user input consisting of the arguments. -
The arguments are tokenized and the respective models of each argument are created.
7.1.1. Student Add command
Implementation
The following is a detailed explanation of the operations which StudentAddCommand
performs.
-
After the successful parsing of user input, the
StudentAddCommand#execute(Model model)
method is called which validates the student defined. -
As student names are unique, if a duplicate student is defined, a
CommandException
is thrown which will not add the defined student. -
The method
Model#addStudent(Student student)
will then be called to add the student. The command box will be reflected with theStudentAddCommand#MESSAGE_SUCCESS
constant and a newCommandResult
will be returned with the success message.If the format or wording of adding a student contains error(s), the behaviour of TeaPet will be that either a unknown command or wrong format error message will be displayed. -
The newly created student is added to the
UniqueStudentList
.
The following activity diagram summarizes what happens when a user executes the student add
command:
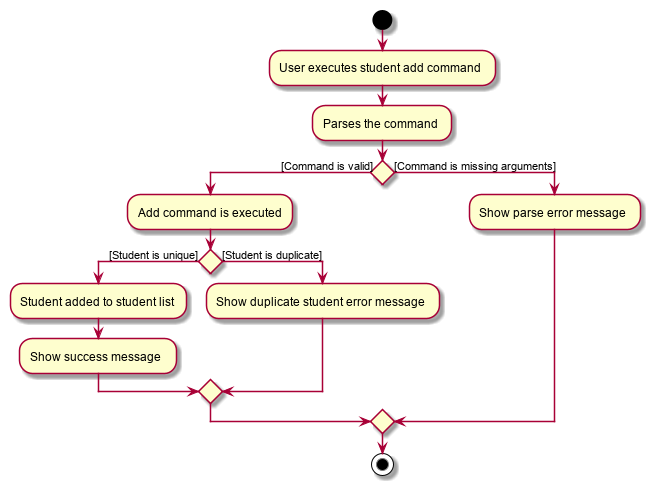
7.1.2. Student Edit command
Implementation
The following is a detailed explanation of the operations which StudentEditCommand
performs.
-
After the successful parsing of user input, the
StudentEditCommand#execute(Model model)
method is called which checks if theIndex
is defined as an argument when instantiating theStudentEditCommand(Index, index, EditStudentDescriptor editStudentDescriptor)
constructor. It uses theStudentEditCommand.EditStudentDescriptor
to create a new edited student. -
A new
Student
with the newly updated values will be created which replaces the existingStudent
object using theModel#setStudent(Student target, Student editedStudent)
method. -
The filtered student list is then updated with the new
Student
with theModel#updateFilteredStudentList(PREDICATE_SHOW_ALL_STUDENTS)
method. -
The command box will be reflected with the
StudentEditCommand#MESSAGE_SUCCESS
constant and a newCommandResult
will be returned with the success message.
7.1.3. Student Delete command
Implementation
The following is a detailed explanation of the operations which StudentDeleteCommand
performs.
-
After the successful parsing of user input, the
StudentDeleteCommand#execute(Model model)
method is called which checks if theIndex
is defined as an argument when instantiating theStudentDeleteCommand(Index index)
constructor.The Index
must be within the bounds of the student list.
-
The
Student
at the specifiedIndex
is then removed from theUniqueStudentList#students
observable list using theModel#deleteStudent(Index index)
method. -
The command box will be reflected with the
StudentDeleteCommand#MESSAGE_SUCCESS
constant and a newCommandResult
will be returned with the success message.
7.1.4. Student Find command
Implementation
The following is a detailed explanation of the operations which StudentFindCommand
performs.
-
After the successful parsing of user input, the
StudentFindCommand#execute(Model model)
method is called which checks if theNameContainsKeywordsPredicate(keywords)
is defined as part of the argument when instantiating theStudentFindCommand(NameContainsKeywordsPredicate predicate)
constructor. -
The
Student
is then searched through theUniqueStudentList#students
list using theModel#hasStudent(Student student)
method to check if theStudent
already exists. If theStudent
does not exist, aStudentNotFoundException
will be thrown and theStudent
will not be displayed. -
The existing
UniqueStudentList#internalList
is then cleared and updated using theModel#updateFilteredStudentList(Predicate predicate)
method. -
A new
CommandResult
will be returned with the success message.
7.1.5. Student Clear command
Implementation
The following is a detailed explanation of the operations which StudentFindCommand
performs.
-
After the successful parsing of the user input, the
StudentClearCommand#execute(Model model)
method is called. -
The
Model#setTeaPet(ReadOnlyTeaPet teaPet)
method is then called which triggers theTeaPet#resetData(ReadOnlyTeaPet newData)
method and creates a brand new student list to replace the old one. -
A new
CommandResult
will be returned with the success message.
Aspect: Command Syntax
-
Current Implementation:
-
Current implementation of the feature follows just the command word syntax For example,
student
.
-
-
Alternatives Considered:
-
We considered using the forward slash
/
before the command word, for example/add
. However, we realise that it is redundant and will make inputs more tedious and confusing for users.
-
Aspect: Command Length:
-
Current Implementation:
-
Commands are shortened as much as possible without much loss in clarity. For example, instead of using
/temperature
, we used/temp
instead to input the students temperature into the application. Although this may be initially unfamiliar to users, it should be easy to pick up and make it less tedious during input.
-
-
Alternatives Considered:
-
We considered using more descriptive arguments such that arguments are clear and succinct. However, this will definitely decrease the user expereince as the command will be too long to type.
-
7.1.6. Import image feature
This feature was included in TeaPet to help teachers easily identify the students using their pictures instead of just names.
This feature utilises the StudentCard#updateImage
method to update the images of students.
The feature comprises of one command namely, DefaultStudentDisplayCommand
-
Updates the student list to show updated images of students and displays the student list.
This is further illustrated in the following sequence diagram below.
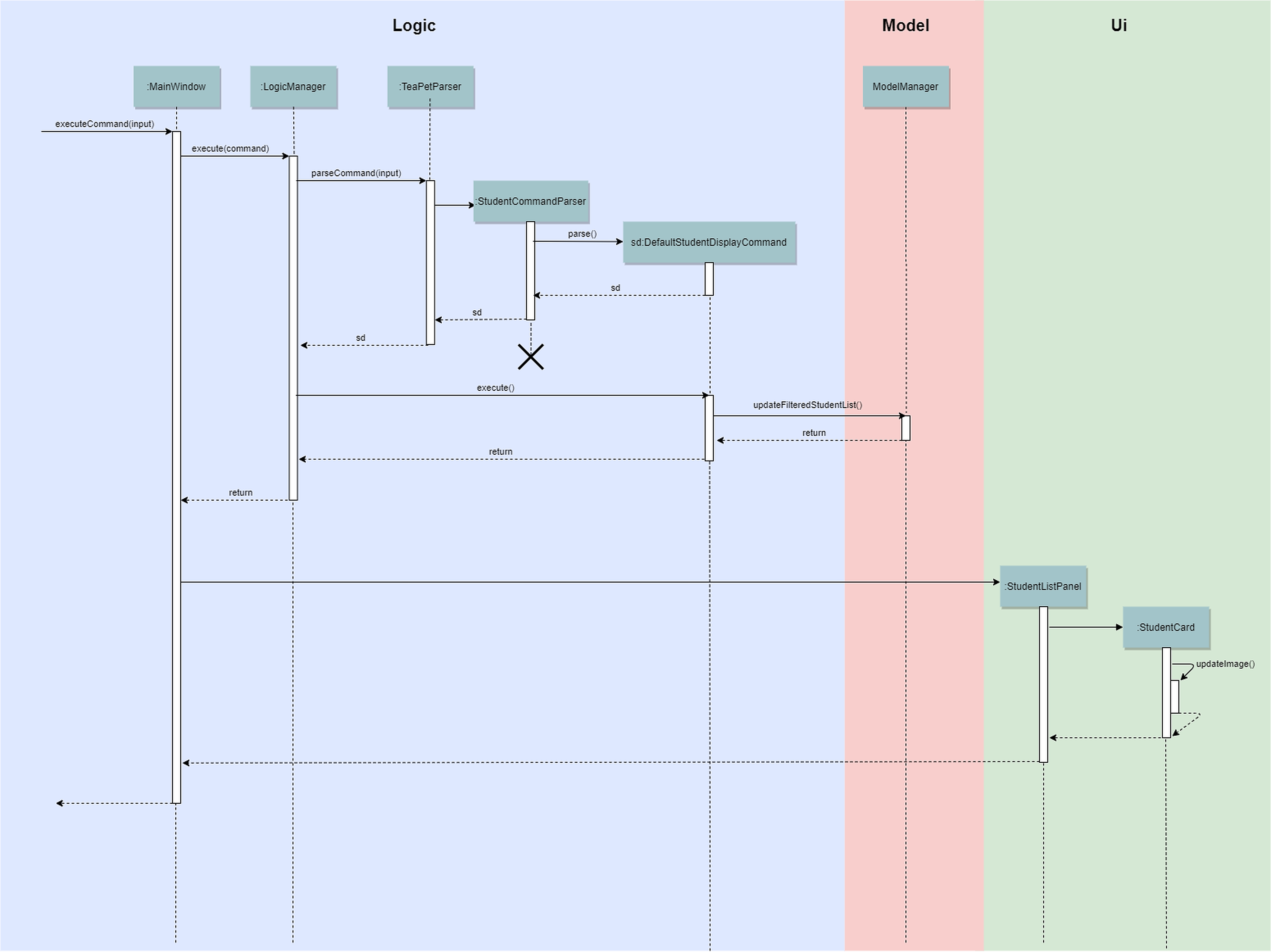
DefaultStudentDisplayCommand
Implementation
The following is a detailed explanation of the operations which DefaultStudentDisplayCommand
performs.
-
After the successful parsing of user input, the
DefaultStudentDisplayCommand#execute(Model model)
method is called. It does not require validation as it does not write into the student list. -
The
StudentCardDefault#updateImage
method is then called which checks the image folder for the required png file and updates the student card.The name of the png file must match the name of the student. -
If any view other than the student list view is showing on the
MainWindow
, theMainWindow#handleDefaultStudent()
method will be called and the student list is now visible on theMainWindow
.
The following activity diagram summarizes what happens when a user executes the student
command:
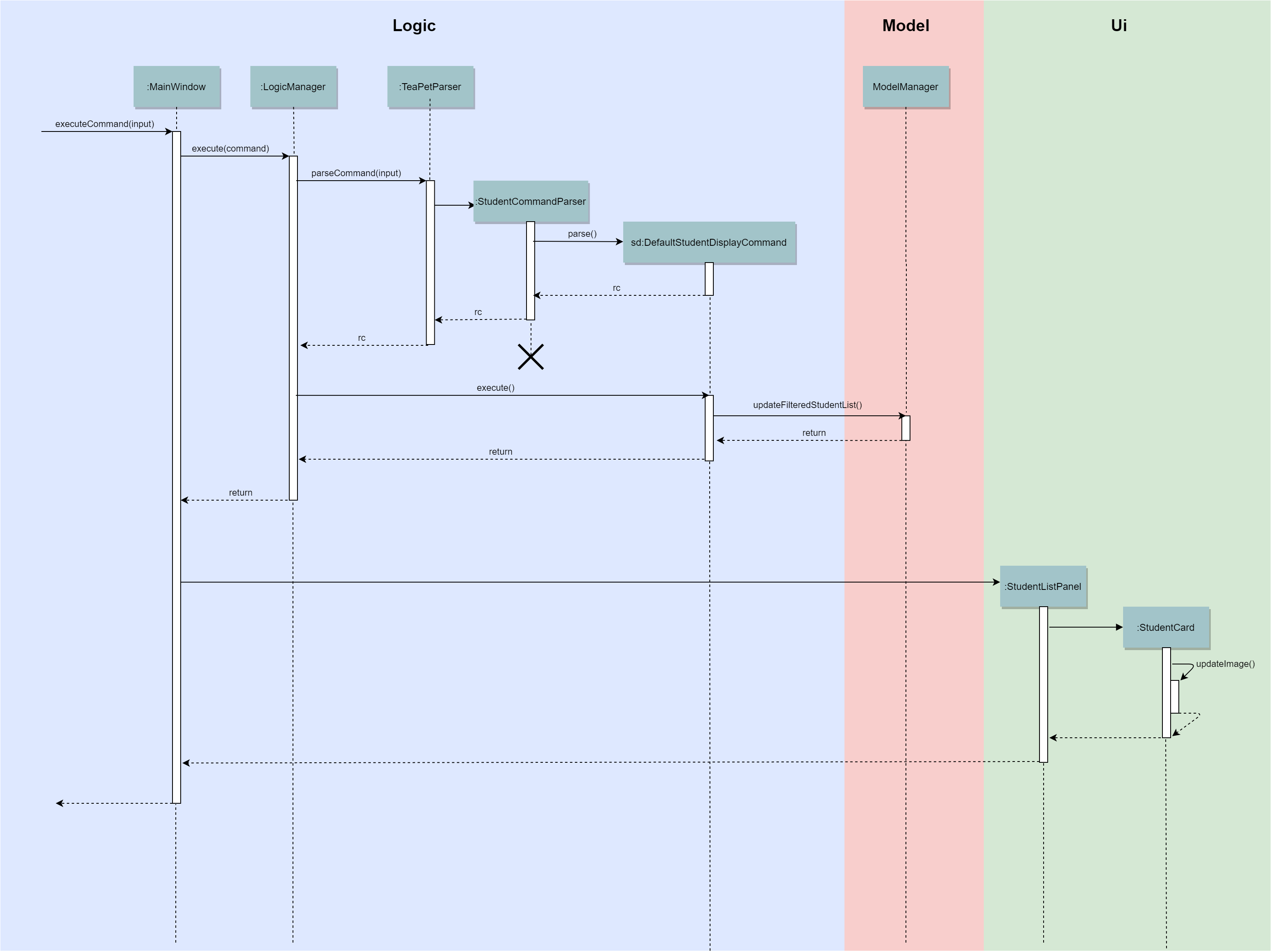
Aspect: Command Syntax
-
Current Implementation:
-
Current implementation of the commands follows the command word syntax, followed by the arguments necessary for execution. For example,
student add/edit/delete/find
.
-
-
Alternatives Considered:
-
We considered using a whole new command,
student refresh
to solely refresh and update images of the students. However, we realised that it would be more convenient for the user if we just add this functionality into thestudent
command instead as it is able to both update the images and display the student list concurrently.
-
7.2. Class administration feature
The class administration feature keeps track of essential student administrative details. The feature comprises of four commands namely.
The structure of the Admin commands are as shown below:
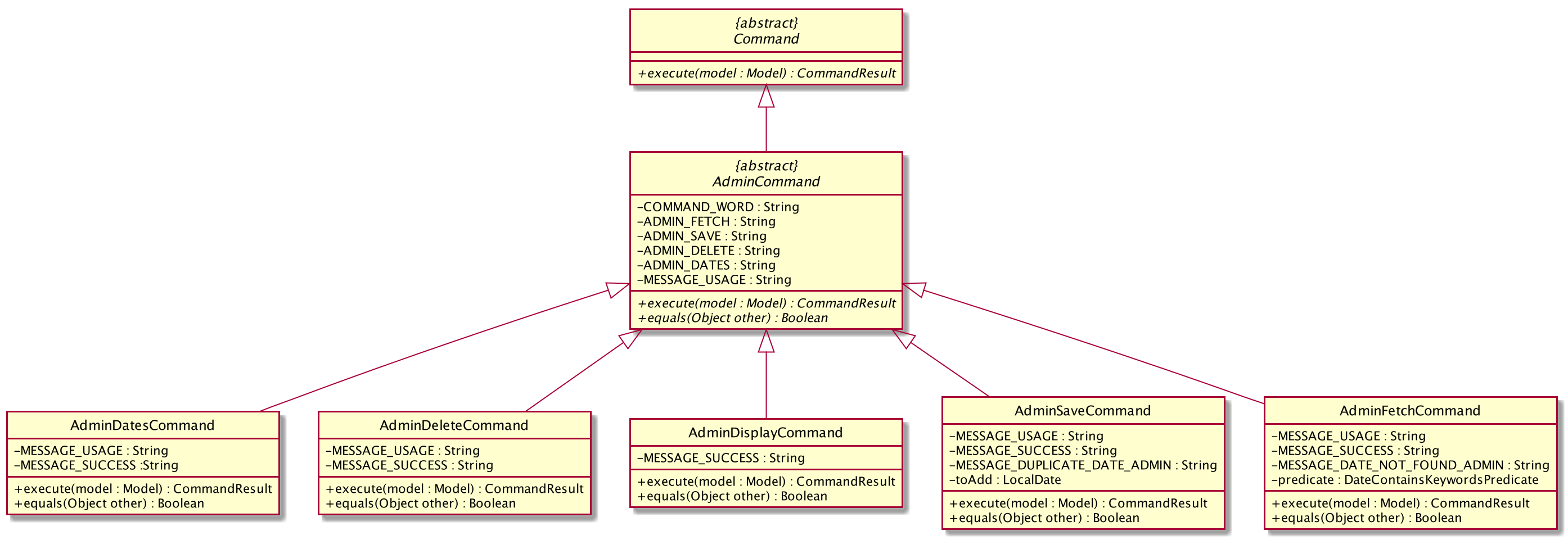
The student administrative feature keeps track of essential student admin details. The feature comprises of commands namely,
-
AdminDisplayCommand
- Displays the most updated class administrative details. -
AdminDatesCommand
- Displays the dates that hold administrative information of the class. -
AdminSaveCommand
- Saves today’s administrative information of the class. -
AdminDeleteCommand
- Deletes the administrative information of the class at the specified date. -
AdminFetchCommand
- Fetches the administrative information of the class at the specified date.
7.2.1. Class Overview
The class diagram below depicts the structure of the Admin Model Component.
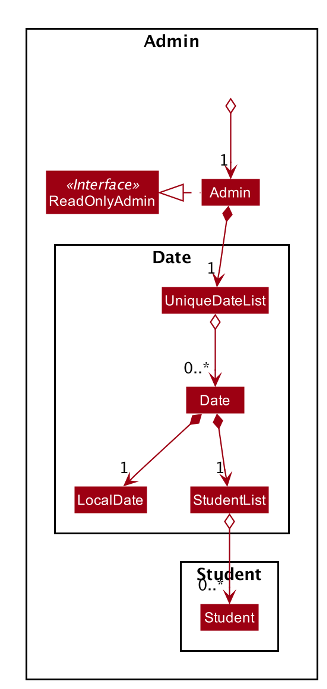
7.2.2. Admin Display Command
Implementation
The following is a detailed explanation of the operations which AdminDisplayCommand
performs.
Step 1. The AdminDisplayCommand#execute(Model model)
method is executed which does not take in any arguments.
Step 2. The method Model#updateFilteredStudentList(PREDICATE_SHOW_ALL_STUDENTS)
will then be called to update the
filtered student list to show all current students in the student list.
If the class list is empty, a blank page will be shown. |
Step 3. The command box will be reflected with the AdminDisplayCommand#MESSAGE_SUCCESS
constant and a new
CommandResult
will be returned with the message.
If the wording of the AdminDisplayCommand contains error(s), an unknown command message will be displayed.
|
7.2.3. Admin Dates Command
Implementation
The following is a detailed explanation of the operations which AdminSaveCommand
performs.
Step 1. The AdminDatesCommand#execute(Model model)
method is executed which does not take in any arguments.
Step 2. The method Model#updateFilteredDateList(PREDICATE_SHOW_ALL_DATES)
will then be called to update the
filtered date list to show all current dates in the date list.
If the date list is empty, a blank page will be shown. |
Step 3. The command box will be reflected with the AdminDatesCommand#MESSAGE_SUCCESS
constant and a new
CommandResult
will be returned with the message.
If the format or wording of the AdminDatesCommand command contains error(s), an unknown command or a wrong format message
will be displayed.
|
7.2.4. Admin Save Command
Implementation
The following is a detailed explanation of the operations which AdminSaveCommand
performs.
Step 1. The AdminSaveCommand#execute(Model model)
method is executed which takes in today’s date as an argument.
Step 2. The method Model#updateFilteredStudentList(PREDICATE_SHOW_ALL_STUDENTS)
will then be called to update the
filtered student list to show all current students in the student list.
Step 3. Sequentially, a date constructor will then called, creating a date object with today’s date and
Model#getFilteredStudentList()
Step 4. The method Model#addDate(Date date)
will then be called to add the date. This will then trigger the
UniqueDateList#addDate(Date toadd)
method, which will throw DuplicateDateException
if the date that is been added
exists, with the duplicate dates error message.
Step 5. The command box will be reflected with the AdminSaveCommand#MESSAGE_SUCCESS
constant and a new
CommandResult
will be returned with the message.
If the format or wording of AdminSaveCommand contains error(s), an unknown command or wrong format error message will be
displayed.
|
The following activity diagram summarizes what happens when a user executes AdminSaveCommand:
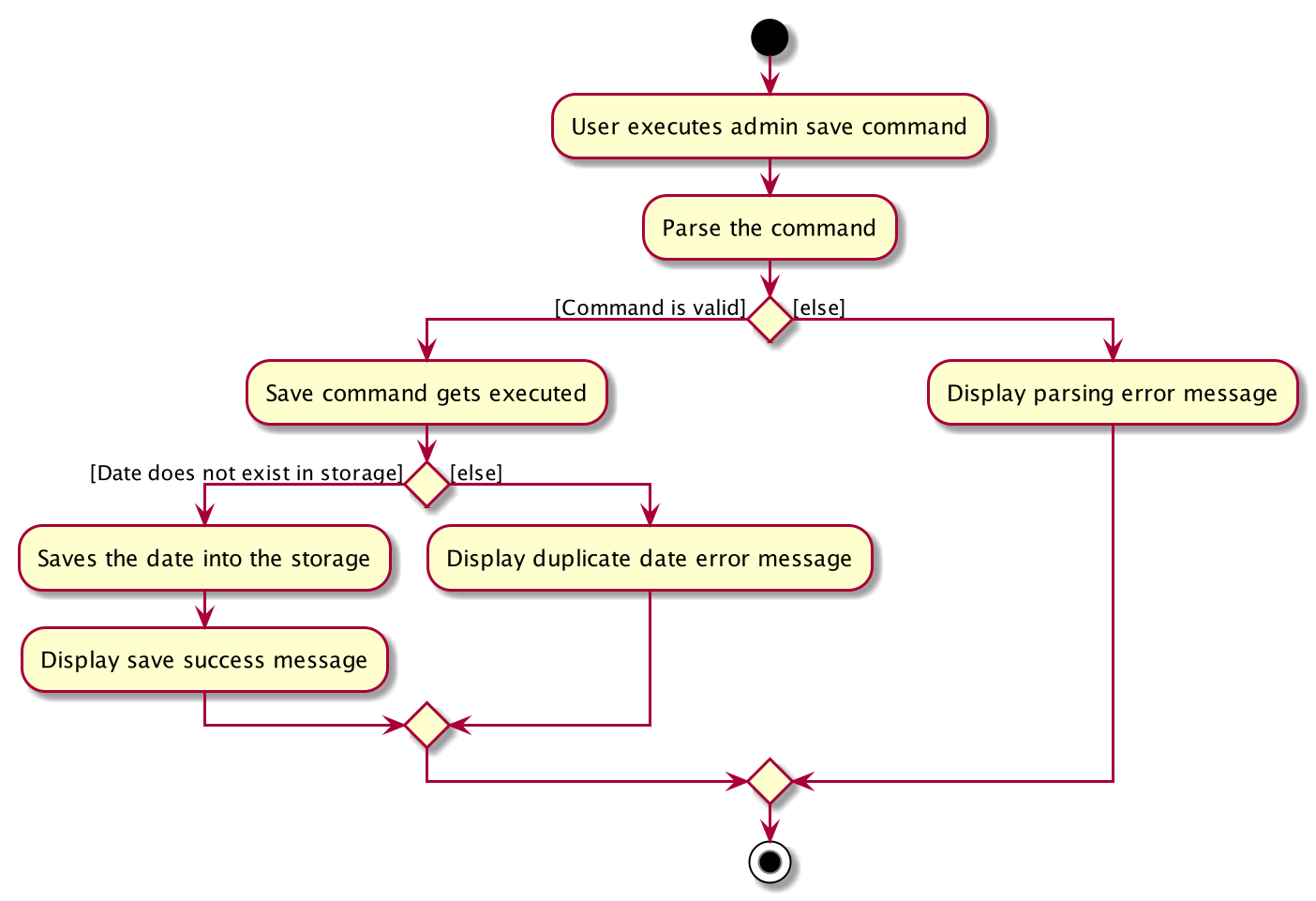
Aspect: Which date to save
-
Current Implementation: Saves the most updated administrative list as today’s date.
-
Pros: Easy to implement and prevents mutation of dates.
-
Cons: The user will be unable to overwrite dates.
-
-
Alternatives Considered: Saves the most updated administrative list as any date.
-
Pros: The user can mutate any dates as he or she wishes.
-
Cons: Hard to implement, and possible accidental mutation of dates.
-
Aspect: Allow overwriting of data
-
Current Implementation: Saving a date that exists in the storage is not allowed.
-
Pros: Easy to implement and prevent accidental mutation of data
-
Cons: Hard to implement.
-
-
Alternatives Considered: Saving a date that exists in the storage is allowed.
-
Pros: User can make necessary changes to the dates where errors exists.
-
Cons: Hard to implement and could result in accidental mutation of dates.
-
7.2.5. Admin Delete Command
Implementation
The following is a detailed explanation of the operations which admin save
performs.
Step 1. The AdminDeleteCommand#execute(Model model)
method is executed which takes in a
DateContainsKeywordsPredicate object as an argument. User input will be parsed first to a DateContainsKeywordsPredicate
object before passing to the`AdminDeleteCommand` constructor.
Date is to be entered in YYYY-MM-DD format, or a ParseException will be thrown and an error message will be displayed. |
Step 2. The method Model#updateFilteredStudentList(DateContainsKeywordsPredicate predicate)
will then be called to
update the filtered date list to show the date that matches the given predicate. If no such date is found after
searching through the UniqueDateList#dates
, a DateNotFoundException will be thrown with an error message displayed.
Step 3. After the date has been found, the method Model*deleteDate(Date target)
will then be called to remove the
specified date from UniqueDateList
.
The following sequence diagram shows how the add operation works:
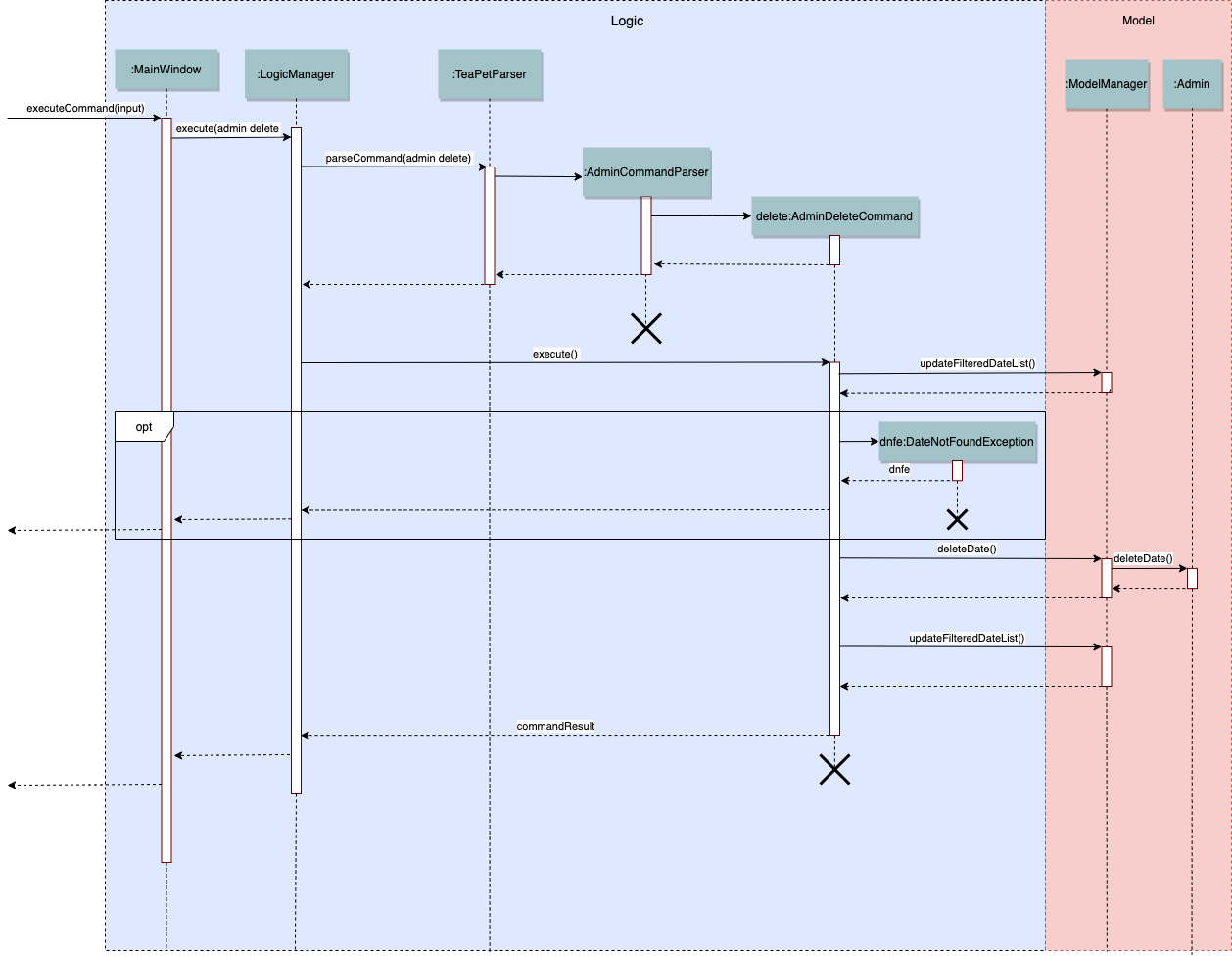
7.2.6. Admin Fetch Command
Step 1. The AdminFetchCommand#execute(Model model)
method is executed which takes in a
DateContainsKeywordsPredicate object as an argument. User input will be parsed first to a DateContainsKeywordsPredicate
object before passing to the`AdminFetchCommand` constructor.
Date is to be entered in YYYY-MM-DD format, or a ParseException will be thrown and an error message will be displayed. |
Step 2. The method Model#updateFilteredStudentList(DateContainsKeywordsPredicate predicate)
will then be called to
update the filtered date list to show the date that matches the given predicate. If no such date is found after
searching through the UniqueDateList#dates
, a DateNotFoundException will be thrown with an error message displayed.
The sequence diagram for admin fetch command is similar to that of admin delete command.
|
7.3. Student Academics feature
This student academics feature stores and tracks the class' academics progress.
The academics feature consists of the following commands namely,
-
AcademicsCommand
- Displays the most updated student academics details. -
AcademicsAddCommand
- Adds a new assessment to the academic list. -
AcademicsEditCommand
- Edits the details of a particular assessment. -
AcademicsDeleteCommand
- Deletes the specified assessment from academics. -
AcademicsSubmitCommand
- Submits students' work to the specified assessment. -
AcademicsMarkCommand
- Marks students' work of the specified assessment. -
AcademicsDisplayCommand
- Displays either homework, exam, or the report of student academics. -
AcademicsExportCommand
- Exports the academics information into a .csv file.
All academics commands share similar paths of execution. The commands when executed, will interface with the methods
exposed by the Model
interface to perform the related operations (See logic component for the general overview).
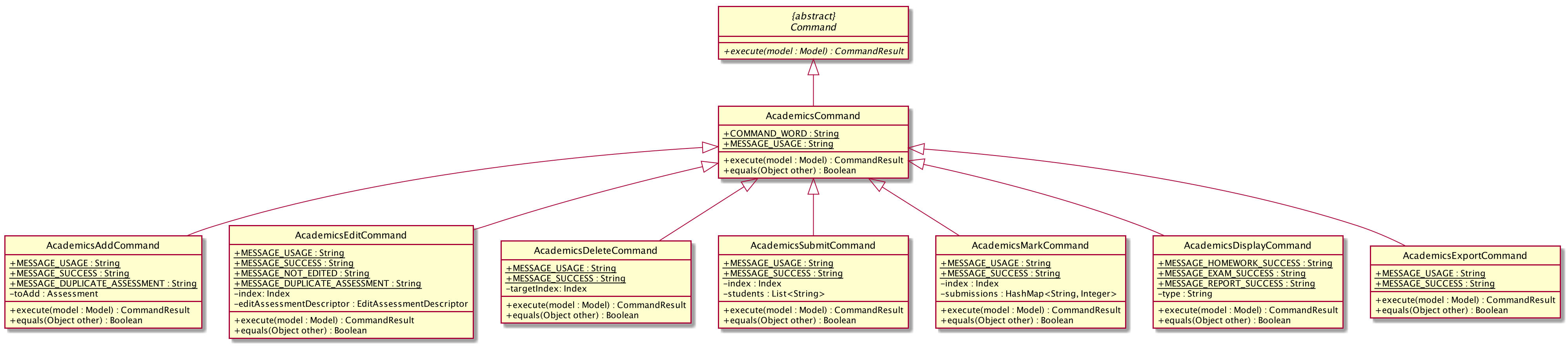
7.3.1. Class Overview
The class diagram below will depict the structure of the Academics Model Component.
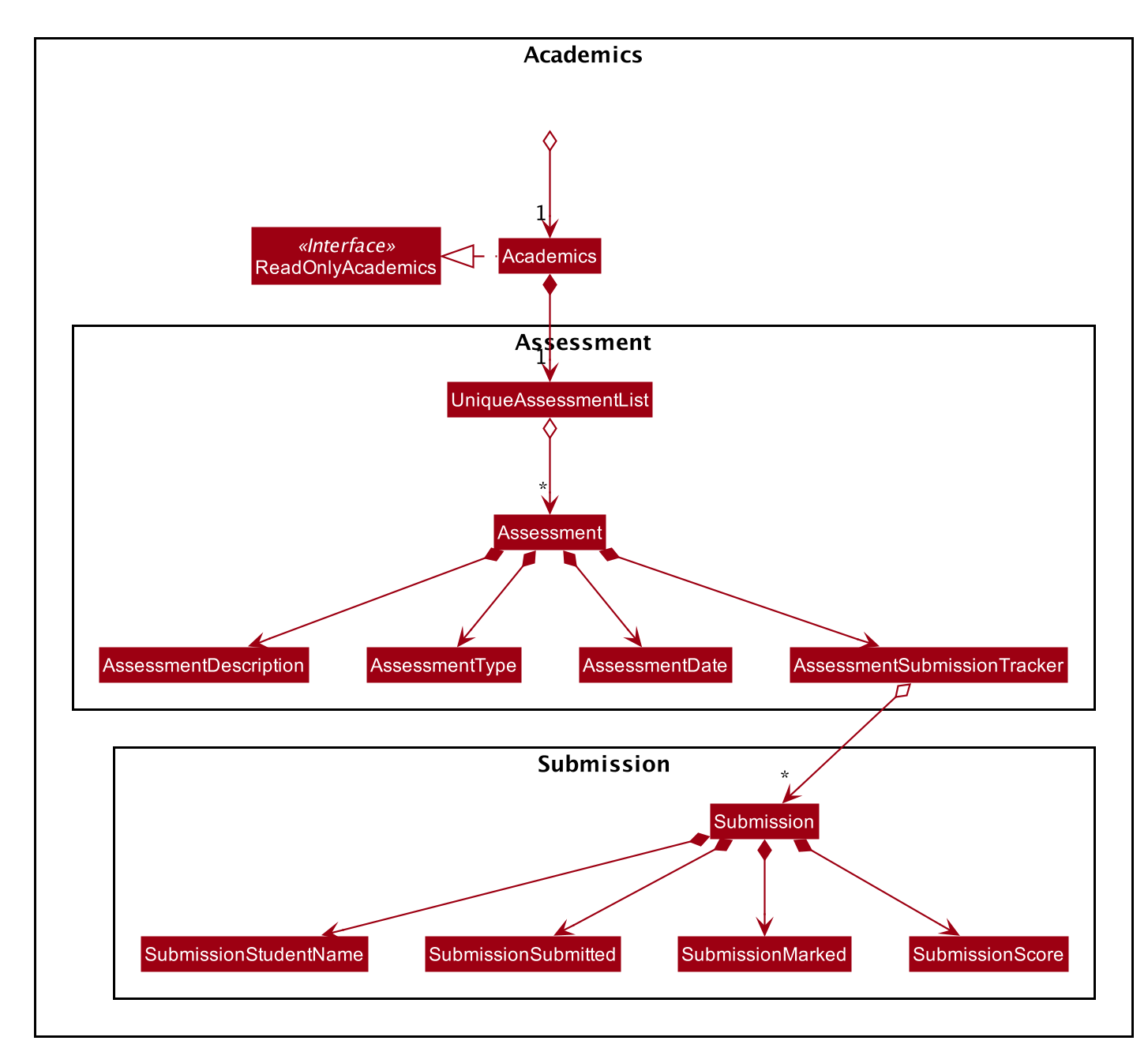
7.3.2. Academics Add Command
Implementation
The following is a detailed explanation of the operations which AcademicsAddCommand
performs.
Step 1. The AcademicsAddCommand#execute(Model model)
method is executed which takes in a necessary assessment
description, type and date.
Format for adding an assessment is academics add desc/ASSESSMENT_DESCRIPTION type/TYPE date/DATE .
|
Step 2. As assessment names should be unique, the Model#hasAssessment(Assessment assessment)
method will check if
the assessment already exists in UniqueAssessmentList#assessments
. If a duplicate assessment is found, a
CommandException
will be thrown.
Step 3. Subsequently, the Model#getFilteredStudentList()
method will then be called, to set the student submission
tracker for the assessment.
Step 4. The method Model#addAssessment(Assessment assessment)
will then be called to add the assessment. The
command box will be reflected with the AcademicsAddCommand#MESSAGE_SUCCESS
constant and a new CommandResult
will be
returned with the message.
If the format or wording of AcademicsAddCommand contains error(s), an unknown command or wrong format error message
will be displayed.
|
The following sequence diagram summarizes what happens when a user executes an AcademicsAddCommand:
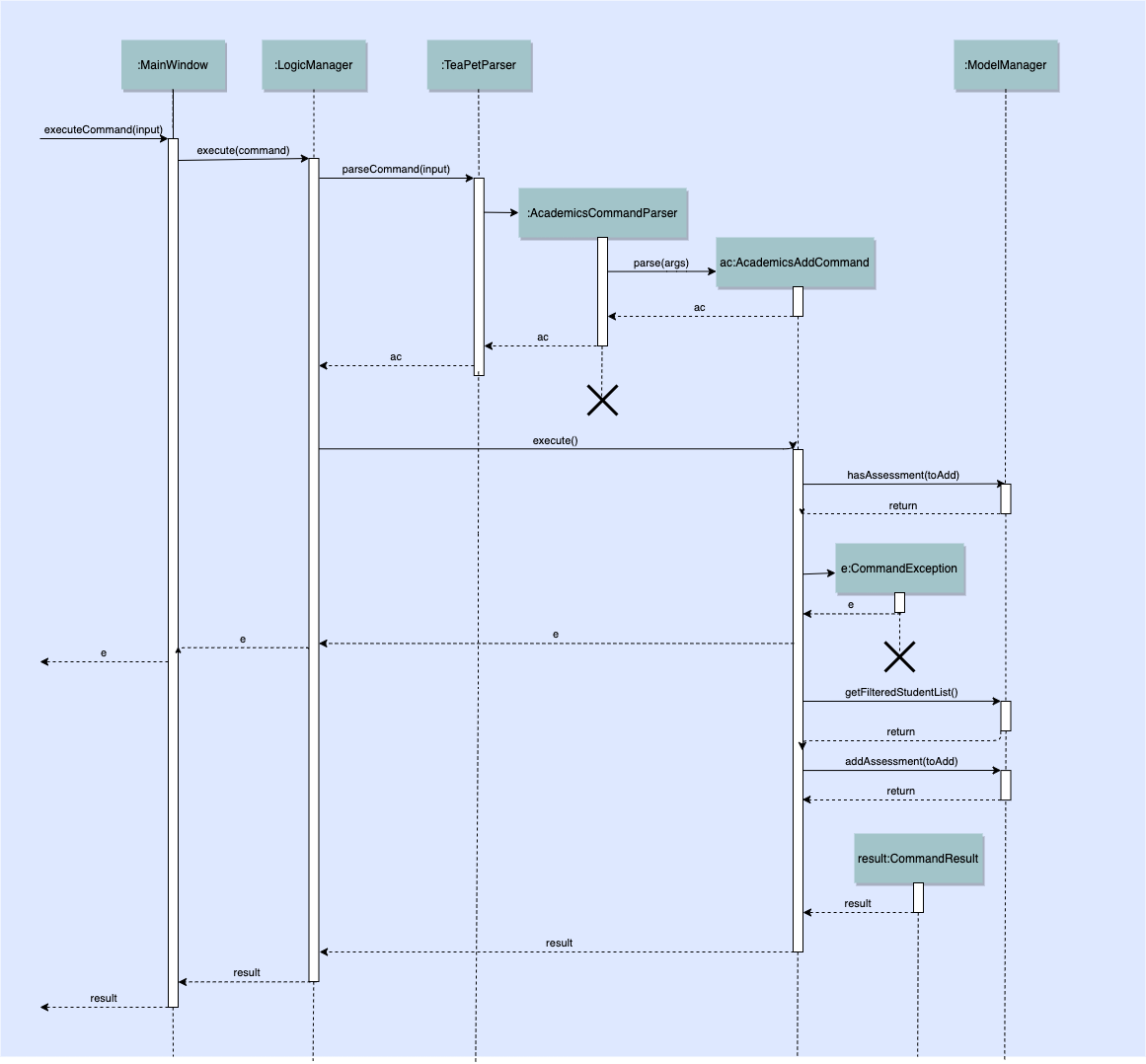
7.3.3. Academics Edit Command
Implementation
The following is a detailed explanation of the operations which AcademicsEditCommand
performs.
Step 1. The AcademicsEditCommand#execute(Model model)
method is executed which edits the details of the specified
assessment. The method checks if the index
defined when instantiating
AcademicsEditCommand(Index index, EditAssessmentDescriptor editAssessmentDescriptor)
is valid. Since it is optional
for users to input fields, the fields not entered will reuse the existing values that are currently stored and defined
in the Assessment
object.
User needs to input at least 1 field of assessment to edit. |
Step 2. A new Assessment
with the newly updated values will be created which replaces the existing Assessment
object using the Model#setAssessment(Assessment target, Assessment editedAssessment)
method. However, if new
assessment results in a duplicate assessment in UniqueAssessmentList#assessments
, a CommandException
will be thrown.
Step 4. The command box will be reflected with the AcademicsEditCommand#MESSAGE_SUCCESS
constant and a new
CommandResult
will be returned with the message.
7.3.4. Academics Delete Command
Implementation
The following is a detailed explanation of the operations which AcademicsDeleteCommand
performs.
Step 1. The AcademicsDeleteCommand#execute(Model model)
method is executed which deletes the assessment at the
specified index. It checks if the Index
is defined as an argument when instantiating the AcademicsDeleteCommand
constructor.
The Index must be within the bounds of UniqueAssessmentList#assessments .
|
Step 2. The Assessment
at the specified Index
is then removed from UniqueAssessmentList#assessments
observable
list using the Model#delete(Assessment assessment)
method.
Step 3. The command box will be reflected with the AcademicsDeleteCommand#MESSAGE_SUCCESS
constant and a new
CommandResult
will be returned with the message.
7.3.5. Academics Submit Command
Implementation
The following is a detailed explanation of the operations which AcademicsSubmitCommand
performs.
Step 1. The AcademicsSubmitCommand#execute(Model model)
method is executed which submits students' work for the
assessment at the specified index. The method checks if the Index
is defined as an argument when instantiating the
AcademicsSubmitCommand
constructor.
The Index must be within the bounds of UniqueAssessmentList#assessments .
|
Step 2. Subsequently, the Model#hasStudentName(String studentName)
method will then check if the given student
exists in UniqueStudentList#students
. Also, Model#hasStudentSubmitted(String studentName)
method checks if the
student has already submitted their work for the specified assessment. If the student does not exist or has already
submitted their work, a CommandException
will be thrown.
Step 3. The students' Submission
will then be submitted to the specified Assessment
using the method
Model#submitAssessment(Assessment assessment, List<String> students)
.
Step 4. The command box will be reflected with the AcademicsSubmitCommand#MESSAGE_SUCCESS
constant and a new
CommandResult
will be returned with the message.
The following activity diagram summarizes what happens when a user executes an AcademicsSubmitCommand:
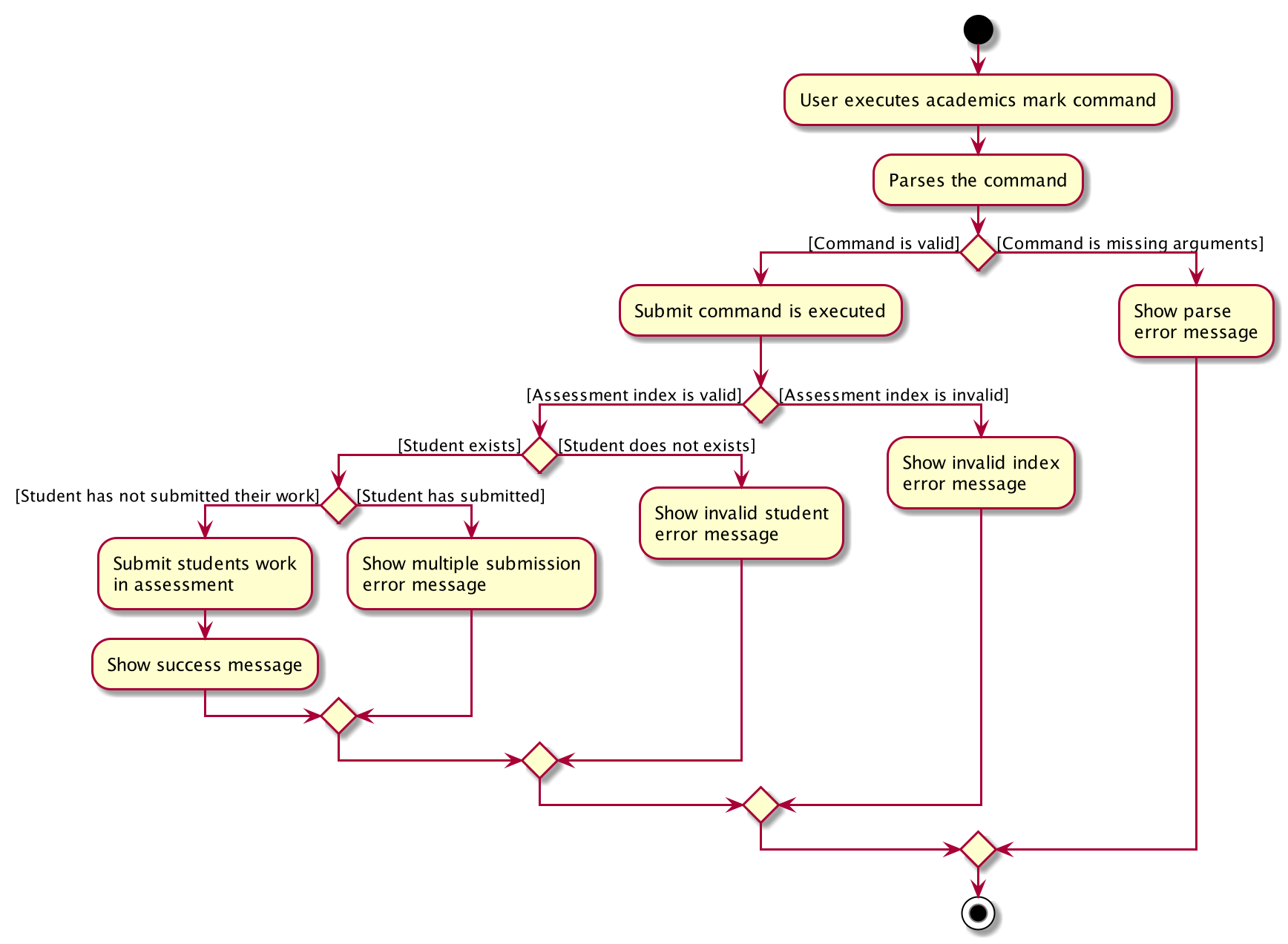
7.3.6. Academics Mark Command
Implementation
The following is a detailed explanation of the operations which AcademicsMarkCommand
performs.
Step 1. The AcademicsMarkCommand#execute(Model model)
method is executed which marks students' work and stores the
students' scores for the assessment at the specified index. The method checks if the Index
is defined as an argument
when instantiating the AcademicsMarkCommand
constructor.
The Index must be within the bounds of UniqueAssessmentList#assessments .
|
Step 2. Subsequently, the Model#hasStudentName(String studentName)
method will then check if the given student
exists in UniqueStudentList#students
. Also, Model#hasStudentSubmitted(String studentName)
method checks if the
student has yet to submit their work for the specified assessment. If the student does not exist or has not submitted
their work, a CommandException
will be thrown. Furthermore, the score should be between 0 and 100 inclusive,
otherwise CommandException
will also be thrown.
Format for marking a students' work is academics mark INDEX stu/STUDENT_NAME-SCORE .
|
Step 3. The students' Submission
will then be marked and its score will be stored in the specified Assessment
using the method Model#markAssessment(Assessment assessment, List<String> students)
.
Step 4. The command box will be reflected with the AcademicsMarkCommand#MESSAGE_SUCCESS
constant and a new
CommandResult
will be returned with the message.
Aspect: Allow submission along with marking
-
Current Implementation: Marking a submission that has not be submitted is not allowed.
-
Pros: Clearer and prevents confusion in data.
-
Cons: Harder to implement and the user will have to submit students' work separately.
-
-
Alternatives Considered: Marking an unsubmitted work will also submit it for the assessment.
-
Pros: The user can just submit students work using the mark command, giving them less to type.
-
Cons: Prone to confusion of submitting and marking commands.
-
Aspect: Allow customizable total score of assessments
-
Current Implementation: Setting the total score for a submission is not allowed. (Total score for all submissions will be 100.)
-
Pros: Easy to implement and maintains uniformity of data.
-
Cons: User cannot set different total scores for assessments and have to grade it to a 100 weightage.
-
-
Alternatives Considered: Setting the total score for a submission is allowed.
-
Pros: User can make set different total scores to different assessments according to its requirements.
-
Cons: Hard to implement and could result in inconsistency of data.
-
7.3.7. Academics Display Command
Implementation
The following is a detailed explanation of the operations which AcademicsDisplayCommand
performs.
Step 1. The AcademicsDisplayCommand#execute(Model model)
method is executed which can either take in no arguments
or a 1 word argument indicating the type of display to show.
Other than the default display (no arguments needed), there are only 3 types of displays: homework , exam , and
report .Format: academics or academics DISPLAY_TYPE
|
Step 2. Depending on the display type, the command box will reflect its respective AcademicsDisplayCommand#MESSAGE_SUCCESS
constant and a new CommandResult
will be returned with the message.
Example. homework
type display will reflect AcademicsDisplayCommand#MESSAGE_HOMEWORK_SUCCESS
If the academics list is empty, a blank page will be shown. |
If the wording of the AcademicsDisplayCommand contains error(s), an unknown command message will be displayed.
|
7.3.8. Academics Export Command
Implementation
The following is a detailed explanation of the operations which AcademicsExportCommand
performs.
Step 1. The AcademicsExportCommand#execute(Model model)
method is executed which exports the content of Academics
into a csv file in the data folder.
Format of the command is: academics export .
|
Step 2. The command box will be reflected with the AcademicsExportCommand#MESSAGE_SUCCESS
constant and a new
CommandResult
will be returned with the message.
Step 3. Subsequently, the CommandResult
will be processed by the MainWindow
in the UI component and generate a
studentAcademics.csv in the data folder of the current directory
7.4. Notes feature
TeaPet application comes with an in-built notes feature, which serves to allow Teachers to record administrative or behavioural information of his/her students. Each note is tagged to a specific student and acts as a lightweight, digital 'Post It'.
The notes feature comprises of 6 main functionalities represented by 6 commands. They are namely:
-
NotesCommand
- Displays all notes and help on the notes feature. -
NotesAddCommand
- Adds a new note into TeaPet. -
NotesEditCommand
- Edits the details of a note in TeaPet. -
NotesDeleteCommand
- Deletes a note in TeaPet. -
NotesFilterCommand
- Filters the list of notes in TeaPet based on user-input keywords. -
NotesExportCommand
- Exports all notes information into a .csv file
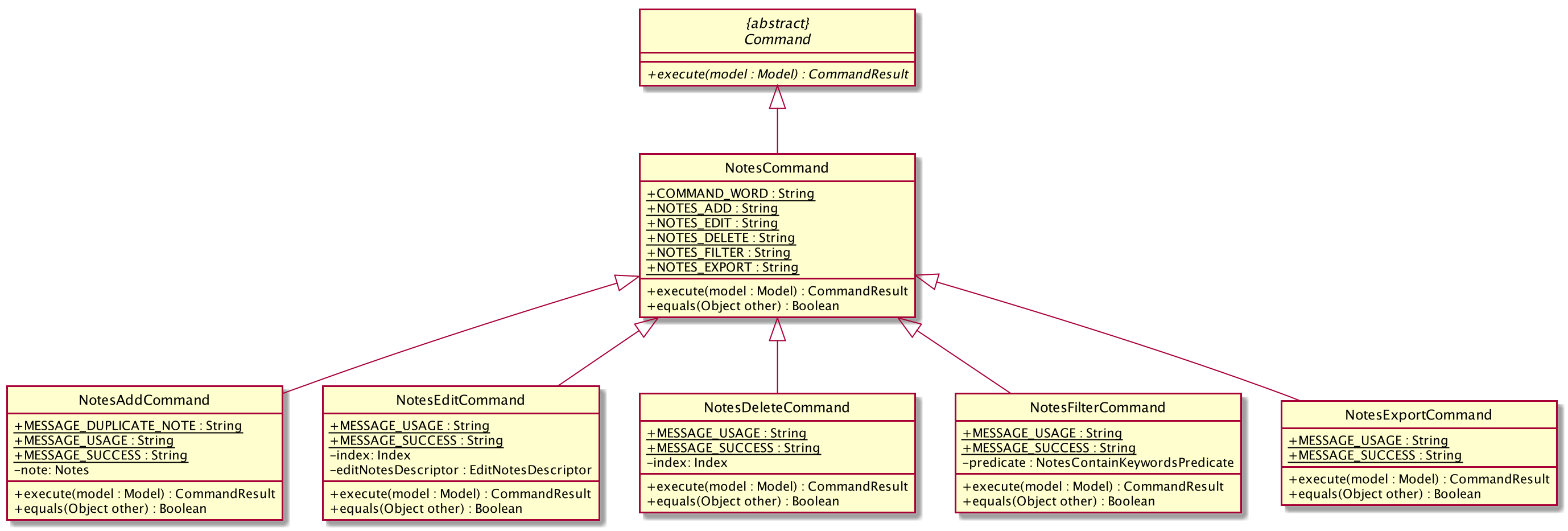
7.4.1. Structure of Notes Class
Notes object is made up of 4 fields. They are namely: Student Name, Content, Priority and DateTime. The class diagram below depicts the structure of the Notes in the Model Component. Please refer to Section 6.4, “Model component” for the higher-level architecture.
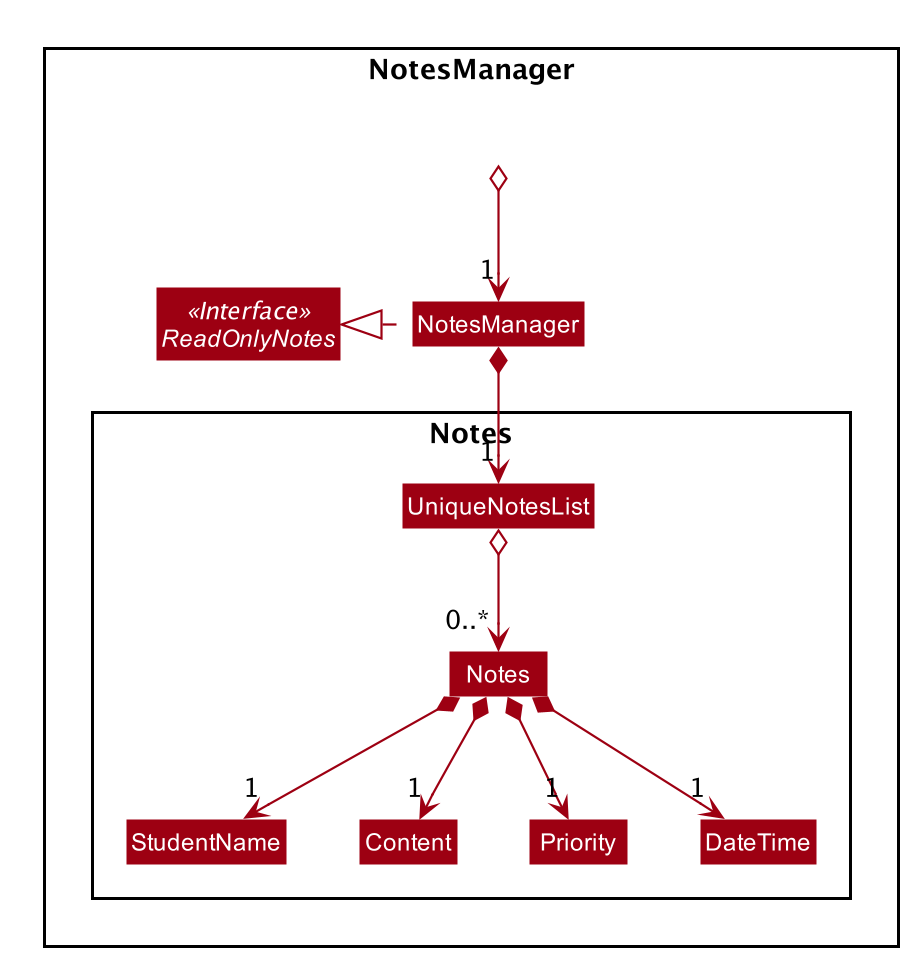
7.4.2. Add Note
The following is a detailed elaboration of how NotesAddCommand
operates.
Format for adding a Note is notes add name/STUDENT_NAME cont/CONTENT pr/PRIORITY .
|
Priority can only be either LOW, MEDIUM or HIGH, case-insensitive. Student must be present in the class list for him/her to be tagged in a note. |
Step 1. The NotesAddCommand#execute(Model model)
method is executed which takes in a necessary student name, content and priority as input.
Step 2. The note is then searched through the UniqueStudentList#students
list using the
Model#hasStudentName(String student)
method to check if the specified student exists. If the student does not exist, the CommandException
will be thrown
with the invalid student error message.
Step 3. The note is then searched through the UniqueNotesList#notes
list using the
Model#hasNote(Notes note)
method to check if the note already exists. If the note exists, the CommandException
will be thrown
with the duplicate note error message.
Step 4. The method Model#addNote(Notes note)
will then be called to add the note. The command box will be reflected with
the NotesAddCommand#MESSAGE_SUCCESS
constant and a new CommandResult
will be returned with the message.
If the format or wording of NotesAddCommand contains error(s), the behaviour of NotesManager will be similar to step 2 and 3, where either a unknown command
or wrong format error message will be displayed.
|
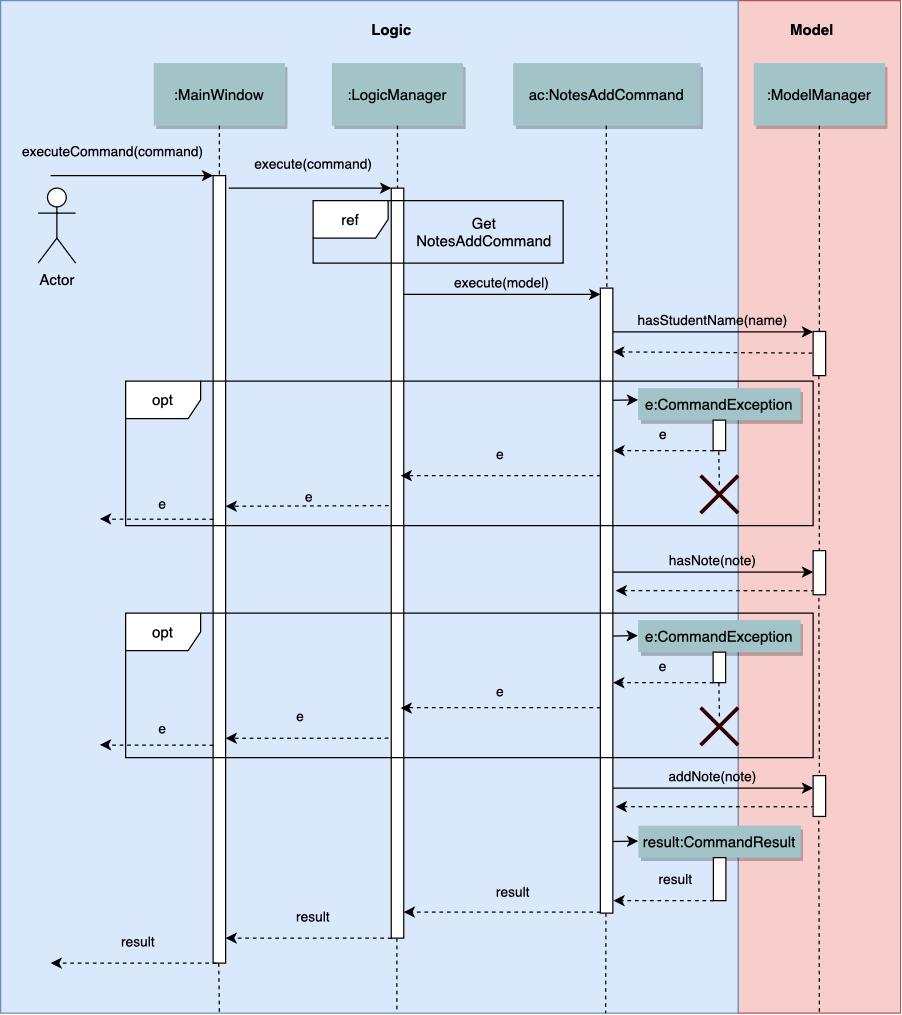
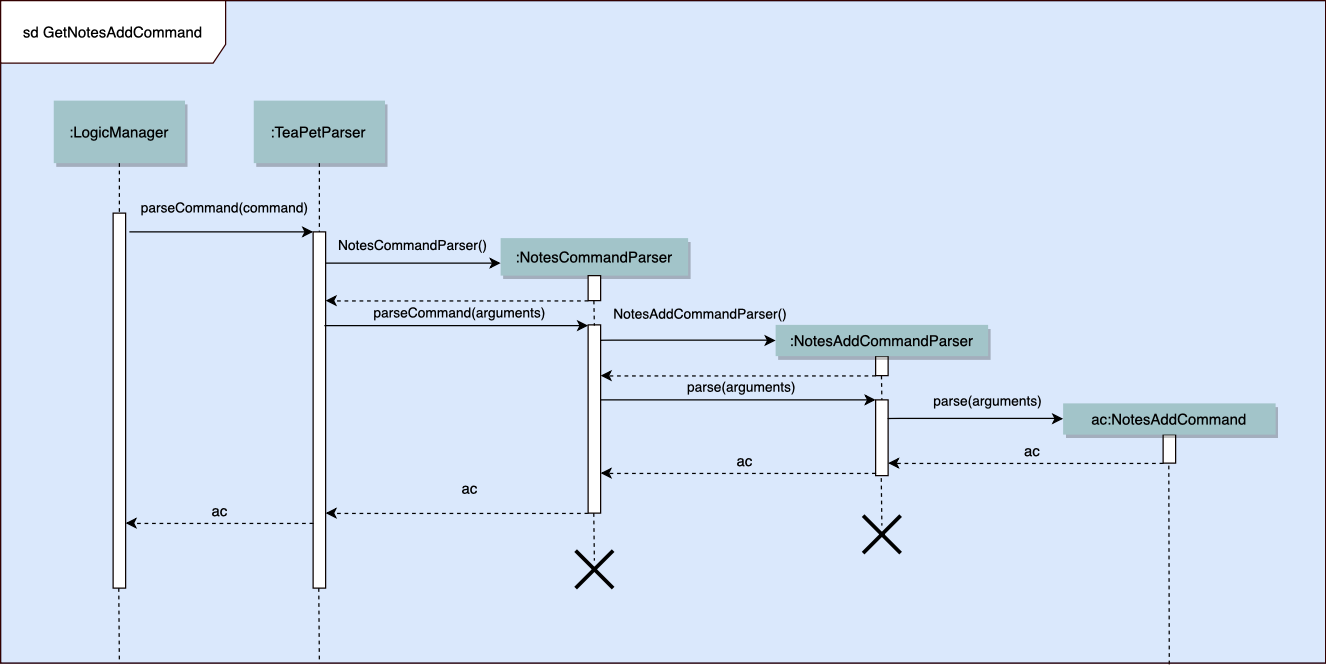
7.4.3. Edit Note
The following is a detailed explanation of the operations which NotesEditCommand
performs.
Format for adding a Note is notes edit INDEX [name/UPDATED_STUDENT_NAME] [cont/CONTENT] [pr/PRIORITY] .
|
Priority can only be either LOW, MEDIUM or HIGH, case-insensitive. Enclosing [] braces indicate optional fields. At least one of the three fields must be present. |
Updated student must be present in the class list for him/her to be tagged in a note. |
Step 1. The NotesEditCommand#execute(Model model)
method is executed which edit attributes of the selected note. The method
checks if the index
defined when instantiating NotesEditCommand(Index index, EditNotesDecriptor editNotesDescriptor)
is
valid. Since it is optional for users to input fields, the fields not entered will reuse the existing values that are currently stored and defined
in the Notes
object.
Step 2. The note is then searched through the UniqueStudentList#students
list using the
Model#hasStudentName(String student)
method to check if the specified student exists. If the student does not exist, the CommandException
will be thrown
with the invalid student error message.
Step 3. A new Notes
with the updated values is created and it is then searched through the UniqueNotesList#notes
list using the
Model#hasNote(Notes note)
method to check if the note already exists. If the note exists, the CommandException
will be thrown
with the duplicate note error message.
Step 4. The newly created Notes
will replace the old one through the Model#setNote(Notes toBeChanged, Notes editedNote
method.
Step 5. The command box will be reflected with the NotesEditCommand#MESSAGE_SUCCESS
constant and a new CommandResult
will be returned with the message.
7.4.4. Delete Note
The following is a detailed explanation of the operations which NotesDeleteCommand
performs.
-
After the successful parsing of user input, the
NotesDeleteCommand#execute(Model model)
method is called which checks if theindex
is defined as an argument when instantiating theNotesDeleteCommand(Index index)
constructor.The Index
must be within the bounds of the list of notes.
-
The
Notes
at the specifiedIndex
is then removed from theUniqueNotesList#notes
observable list using theModel#deleteNote(Index index)
method. -
The command box will be reflected with the
NotesDeleteCommand#MESSAGE_SUCCESS
constant and a newCommandResult
will be returned with the success message.
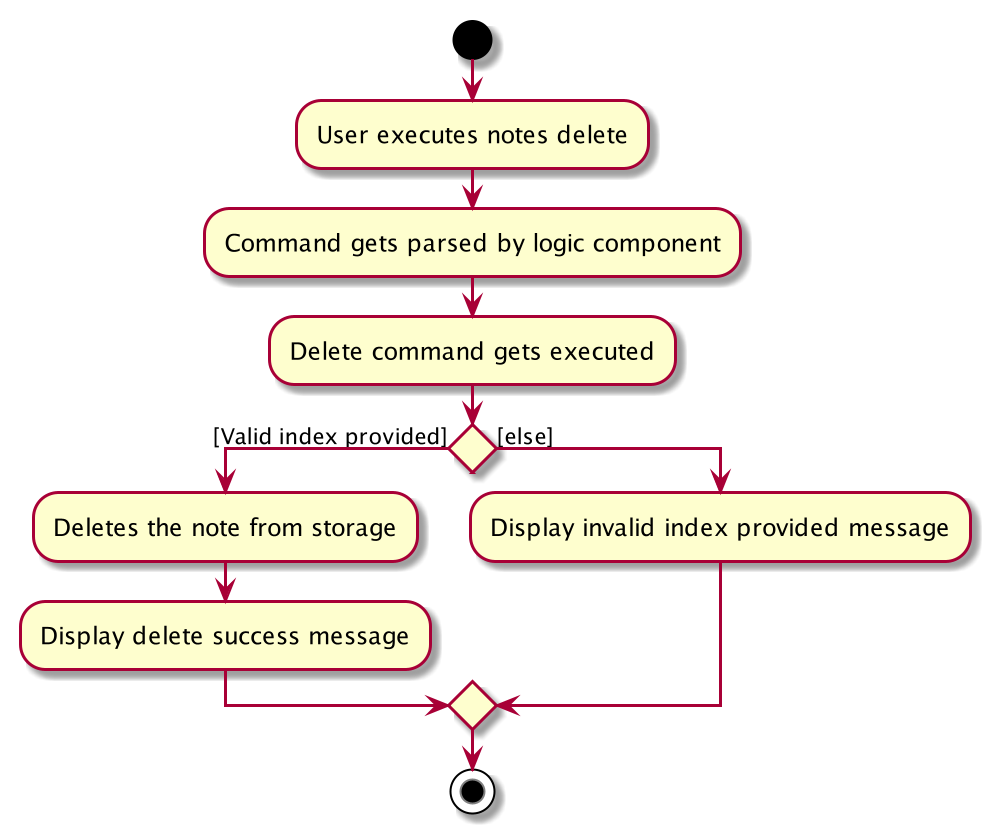
7.4.5. Filter Notes
The following is a detailed explanation of the operations which NotesFilterCommand
performs.
-
After the successful parsing of user input, the
NotesFilterCommand#execute(Model model)
method is called which checks if theNotesContainsKeywordsPredicate(keywords)
is defined as part of the argument when instantiating theNotesFilterCommand(NotesContainsKeywordsPredicate predicate)
constructor. -
The
Notes
objects are then searched through theUniqueNotesList#notes
list, checking for presence of any keyword given by the user. -
The existing
UniqueNotesList#internalList
is then cleared and updated using theModel#updateFilteredNotesList(Predicate predicate)
method. -
A new
CommandResult
will be returned with the success message.
7.4.6. Export Notes
The following is a detailed explanation of the operations which NotesExportCommand
performs.
-
After the successful parsing of user input, the
NotesExportCommand#execute(Model model)
method is called. -
The command box will be reflected with the
NotesExportCommand#MESSAGE_SUCCESS
constant and a newCommandResult
will be returned with the success message. -
The
MainWindow
of the UI component will process theCommandResult
and create a studentNotes.csv in the data folder of the current directory.
Design Considerations
-
Current Implementation: Intuitive, simple syntax and user-friendly
-
Pros: It is easy for the Teacher to use the feature.
-
Cons: Not as powerful and less utility for advanced users.
-
-
Alternatives Considered: Many additional fields including special tags, reminders, etc.
-
Pros: Powerful, many interesting features that advanced users can use.
-
Cons: It contradicts with the initial goal of the Notes feature which is to enable quick and easy note-taking.
-
7.5. Personal Schedule feature
The Personal Schedule feature enables teachers to record down their events in their personal scheduler, which will be sorted accordingly to date and time. Different view modes are available to enable the teachers to easily view their schedule.
This feature is built based on the Jfxtras iCalendarAgenda library. The iCalendarAgenda object is used on the UI side to render VEvents. The VEvent object takes in data such as event name, start date time, end date time, recurrence of events, etc.
VEvent object is used primarily throughout the feature as it is the required object type for the iCalendarAgenda library. Hence, at the storage level, the Event objects are mapped to VEvents for reading purposes and vice versa for saving purposes. |
The Personal Schedule feature allows the user to track and manage their events. The schedule feature consists of eight commands namely:
-
EventDisplayCommand
- Displays all events in the current week. -
EventAddCommand
- Creates a new event in the scheduler. -
EventEditCommand
- Edits the details of an event in the scheduler. -
EventDeleteCommand
- Deletes the specified event from the scheduler. -
EventIndexCommand
- Obtain the index of the specified event. -
EventIndexAllCommand
- Obtain all the indexes of all the events in the scheduler. -
EventViewCommand
- Changes the view mode of the scheduler. -
EventExportCommand
- Exports the event details into an .ics file.
7.5.1. Class Overview
The class diagram below shows the structure of Event classes in the Model
. Notice how the EventHistory
class depends
on the Event
class in its constructor but only has a VEvent
attribute. This is because an Event
object will always be
mapped to a VEvent
within the EventHistory
class. Some methods of EventHistory
has been omitted for brevity as they are
mostly VEvent
based, which then again highlights that the interactions with the Logic
and UI
components are mostly done
using the VEvent
type class. Only the Storage
component works with Event
type class.
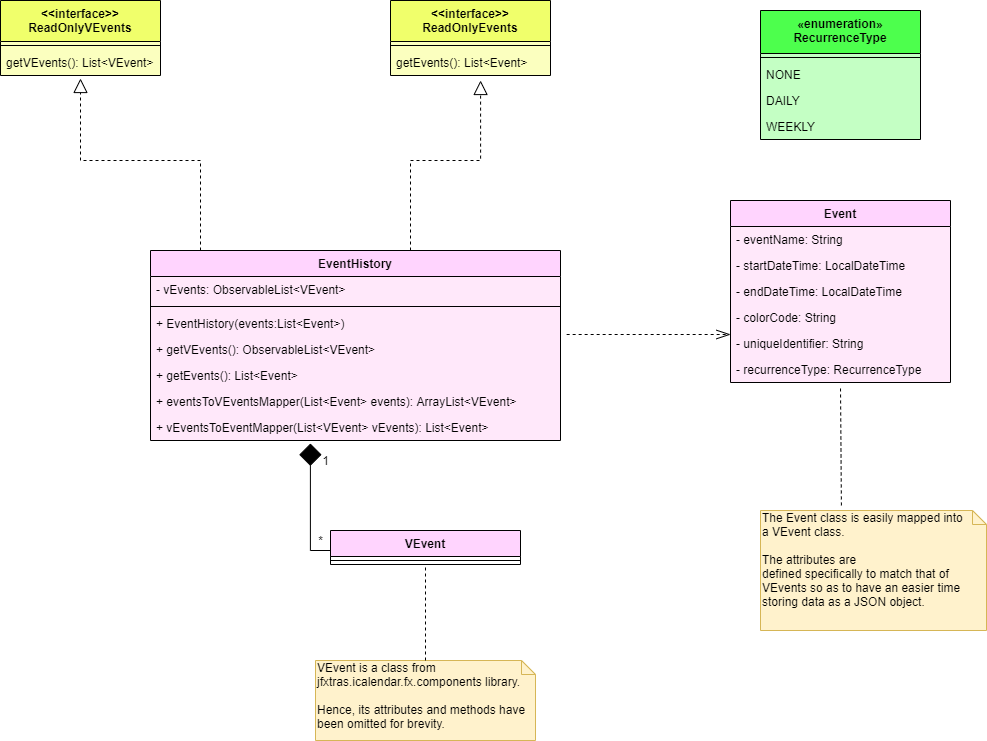
7.5.2. Schedule Display Command
Implementation
The following is a detailed explanation of the operations which EventDisplayCommand
performs.
Format for displaying the schedule is schedule .
|
Step 1: The EventDisplayCommand#execute(Model model)
method is executed and it updates the current model using the method
Model#setEventScheduleLocalDateTime(LocalDateTime localDateTime)
. LocalDateTime#now()
is used to obtain the current LocalDateTime
and used as the parameter in Model#setEventScheduleLocalDateTime(LocalDateTime localDateTime)
.
Step 2: The EventSchedulePrefs#localDateTime
is then updated with the current LocalDateTime
.
Step 3: The CommandBox will be reflected with the EventDisplayCommand#MESSAGE_SUCCESS
constant and
a new CommandResult will be returned with the message.
Aspect: Product cohesiveness
-
Current Implementation:
-
schedule
-
Our team chose to use the one-word command
schedule
as the rest of our features also use one-word commands. This is so that our features look like one integrated product and not an application with several features.
-
-
Alternatives Considered:
-
schedule view
-
If this was the implementation that we used, it can get quite confusing to other users as to why the
academics
command works to change the scene to display the academics whilst to look at the schedule, one must enter inschedule view
.
-
7.5.3. Schedule Add Command
Implementation
The following is a detailed explanation of the operations which EventAddCommand
performs.
Format for adding an event to the schedule is schedule add eventName/EVENT_DESCRIPTION startDateTime/YYYY-MM-DDTHH:MM
endDateTime/YYYY-MM-DDTHH:MM recur/RECUR_DESCRIPTION color/COLOR_CODE
|
RECUR_DESCRIPTION can only take the values none , daily or weekly .
|
COLOR_CODE can only take the values 1 -23 .
|
Step 1: The EventAddCommand#execute(Model model)
method is called which validates if the VEvent
object from
the parser is valid.
Step 2: The method Model#addVEvent(VEvent vEvent)
is then called which adds the new VEvent
to the EventHistory
.
The VEvent
is validated to check if it is unique using the EventUtil#isEqualVEvent(VEvent vEvent)
method.
Step 3: If the event is invalid, a CommandException
will be thrown with an error message.
Step 4: The CommandBox will be reflected with the formatted EventAddCommand#MESSAGE_SUCCESS
constant with the summary of the VEvent
and
a new CommandResult will be returned with this same formatted message.
The following activity diagram summarizes what happens when a user executes the schedule add
command:
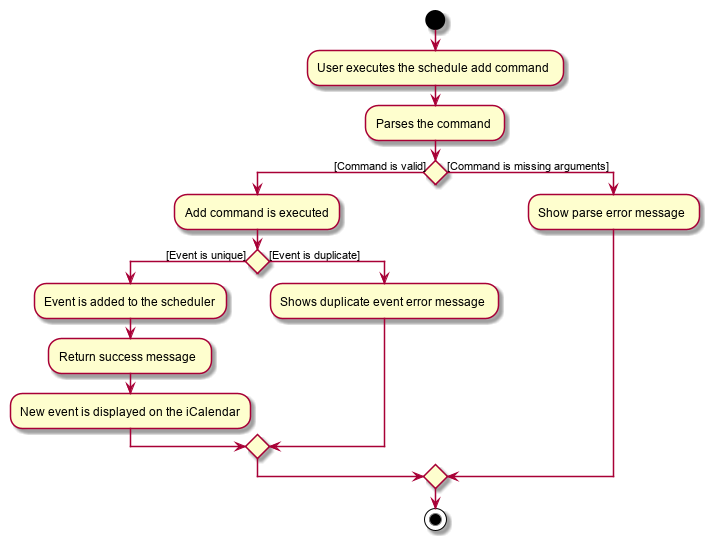
Aspect: Command Clarity
-
Current Implementation:
-
schedule add eventName/Consultation startDateTime/2020-04-08T09:00 endDateTime/2020-04-08T11:00 recur/none color/13
-
We currently have full names for prefixes such as eventName/ instead of name/, as well as slightly lengthier prefixes such as startDateTime/ and endDateTime/. Although this may be slightly more tedious, we believe that it is clearer as there are other very similar prefixes in our other features such as name/ and date/.
-
-
Alternatives Considered:
-
schedule add name/Consultation startDateTime/2020-04-08T09:00 endDateTime/2020-04-08T11:00 recur/none color/13
-
By doing this, users may be confused as the Academics feature, Student feature and Notes feature require name as a prefix as well. Furthermore, the name required here is not the name of the student but the name of the event.
-
7.5.4. Schedule Edit Command
Implementation
The following is a detailed explanation of the operations which EventEditCommand
performs.
Format for editing an event in the schedule is schedule edit INDEX [eventName/EVENT_DESCRIPTION] [startDateTime/YYYY-MM-DDTHH:MM]
[endDateTime/YYYY-MM-DDTHH:MM] [recur/RECUR_DESCRIPTION] [color/COLOR_CODE]
NOTE: INDEX is the index of the event. Which can be obtained by the indexGet Command. See below for more information.
|
RECUR_DESCRIPTION can only take the values none , daily or weekly .
|
COLOR_CODE can only take the values 1 -23 .
|
All fields are optional, however at least one field needs to be entered to edit. |
Step 1: The EventEditCommand#execute(Model model)
method is executed which edits the details of the specified
VEvent
in the model. This method checks if the index
defined when instantiating
EventEditCommand(Index index, EditVEventDescriptor editVEventDescriptor)
is valid. This method also checks if
the editVEventDescriptor
is valid.
Since the fields are optional, the fields not entered by the user will reuse the existing values that are currently stored and defined
in the VEvent
object.
Index must be a positive number greater than zero. |
At least 1 field of event needs to be provided to editVEventDescriptor to edit.
|
Step 2: A new VEvent
with the newly updated values will be created which replaces the existing VEvent
object in the current model using the Model#setVEvent(Index index, VEvent vEvent);
method. The values input to editVEventDescriptor
are then validated and if the values are not valid, an IllegalValueException
will be thrown.
Step 3: The newly created VEvent is then validated. If the newly created VEvent is the same as any of the events in the scheduler
or it has not been edited, a CommandException
will be thrown.
Step 4: The new created VEvent
is then mapped to an Event and the LocalDateTime
of this VEvent
is used to update
EventSchedulePrefs#localDateTime
using the method Model#setEventScheduleLocalDateTime(LocalDateTime localDateTime)
.
Step 5: EventEditCommand#generateSuccessMessage(VEvent vEvent)
formats the success message with
EventEditCommand#MESSAGE_EDIT_EVENT_SUCCESS
, the Index
of the event and the VEvent
.
Step 6: The command box will be reflected with the success message and a new
CommandResult
will be returned with the message.
7.5.5. Schedule Delete Command
Implementation
The following is a detailed explanation of the operations which EventDeleteCommand
performs.
Format for deleting an event in the schedule is schedule delete INDEX .
|
INDEX is the index of the event. Which can be obtained by the indexGet Command. See below for more information. |
Step 1: The EventDeleteCommand#execute(Model model)
method is executed which deletes the specified
VEvent
in the model. This method checks if the targetIndex
defined when instantiating
EventDeleteCommand(Index targetIndex)
is valid.
Index must be a positive number greater than zero and within the bounds of the number of events in the scheduler. |
Step 2: The VEvent
at the specified targetIndex
is then removed from EventHistory#VEvents
using the Model#delete(Index index) method.
Step 3: EventDeleteCommand#generateDeleteSuccessMessage(VEvent vEvent)
formats the success message with
EventDeleteCommand#MESSAGE_SUCCESS
and the VEvent
.
Step 4: The command box will be reflected with the success message and a new
CommandResult
will be returned with the message.
The following sequence diagram will provide a clearer picture on how the EventDeleteCommand works.
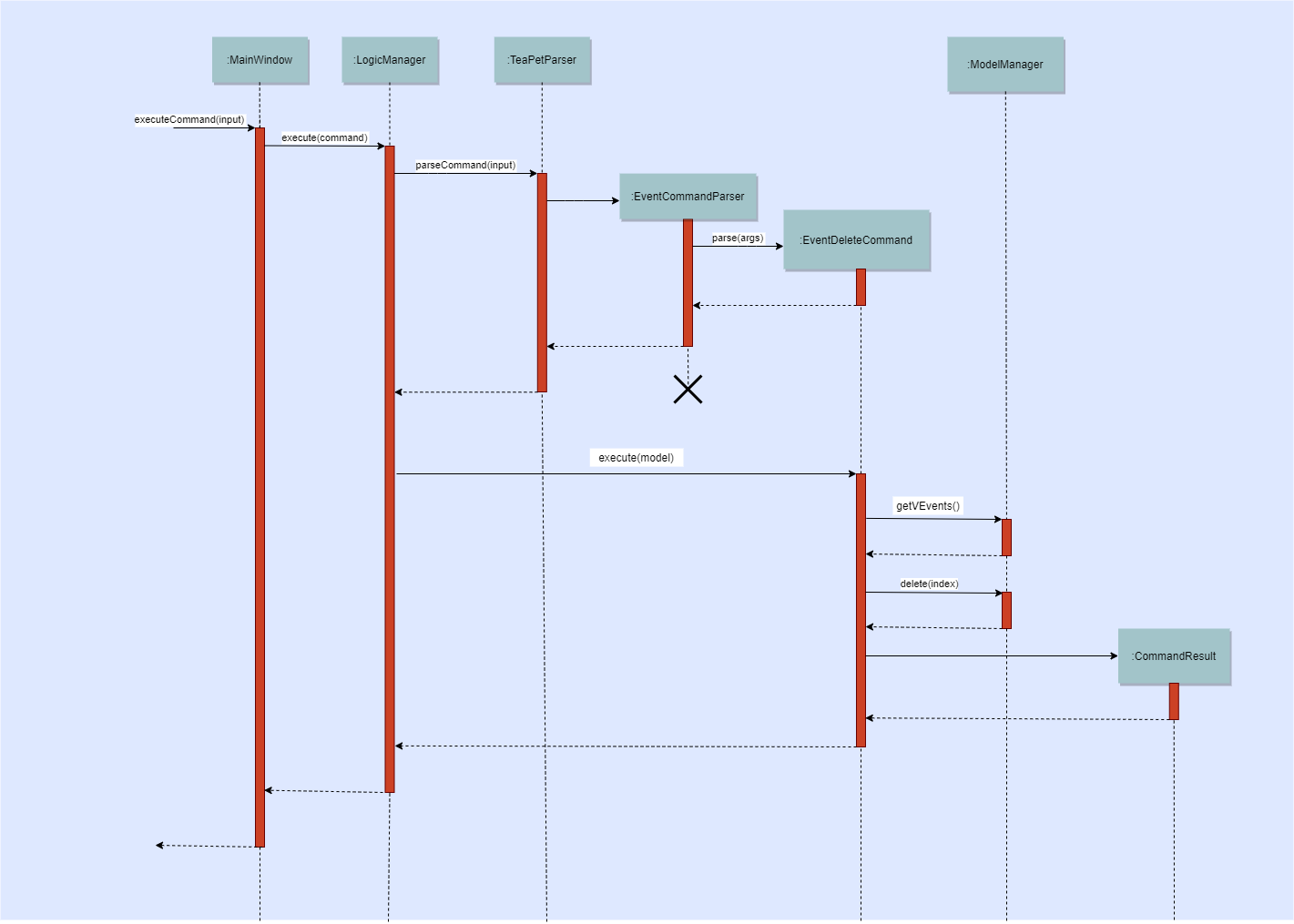
7.5.6. Schedule Index Command
Implementation
The following is a detailed explanation of the operations which EventIndexCommand
performs.
This command returns the index of the Event(s) with same EVENT_DESCRIPTION as the eventName
input from the user.
If there are no Events with the same EVENT_DESCRIPTION, the Event with the most similar EVEnT_DESCRIPTION, calculated
using the Levenshtein Distance formula, will then be suggested.
Format for getting the index of an event in the schedule is schedule indexGet/EVENT_DESCRIPTION .
|
EVENT_DESCRIPTION is the name of the event.
|
Step 1: The EventIndexCommand#execute(Model model)
method is executed which obtains the index of the specified
VEvent
in the model. This method first obtains the current list of Pair
of Index
and VEvents
in EventHistory#vEvents
using
Model#searchVEvents(String eventName)
.
Step 2: The current list of Events is then validated.
-
If the list of Events is empty, the method will find the most similar
Event
inEventHistory#vEvents
based on theeventName
entered when when instantiatingEventIndexCommand(String eventName)
. The foundVEvent
is then mapped to an Event and theLocalDateTime
of thisVEvent
is used to updateEventSchedulePrefs#localDateTime
using the methodModel#setEventScheduleLocalDateTime(LocalDateTime localDateTime)
.VEventNotFoundException
is thrown when there are no events in the schedule at all. -
If the list of Events is not empty, The first
VEvent
of the list of Events is then mapped to an Event and theLocalDateTime
of thisVEvent
is used to updateEventSchedulePrefs#localDateTime
using the methodModel#setEventScheduleLocalDateTime(LocalDateTime localDateTime)
.
Step 3: EventIndexCommand#generateSuggestionMessage(Pair<Index, VEvent> resultVEventPair)
or EventIndexCommand#generateResultMessage(List<Pair<Index, VEvent>> pairVEventIndexList)
will then format the message according to whether there the list of Events is empty.
-
If the list of Events is empty, the method
EventIndexCommand#generateSuggestionMessage(Pair<Index, VEvent> resultVEventPair)
will be called. -
If the list of Events is not empty, the method
EventIndexCommand#generateResultMessage(List<Pair<Index, VEvent>> pairVEventIndexList)
will be called.
Step 4: The command box will be reflected with the message and a new
CommandResult
will be returned with the message.
The following Activity Diagram will provide a clearer picture on how the EventIndexCommand is supposed to run.
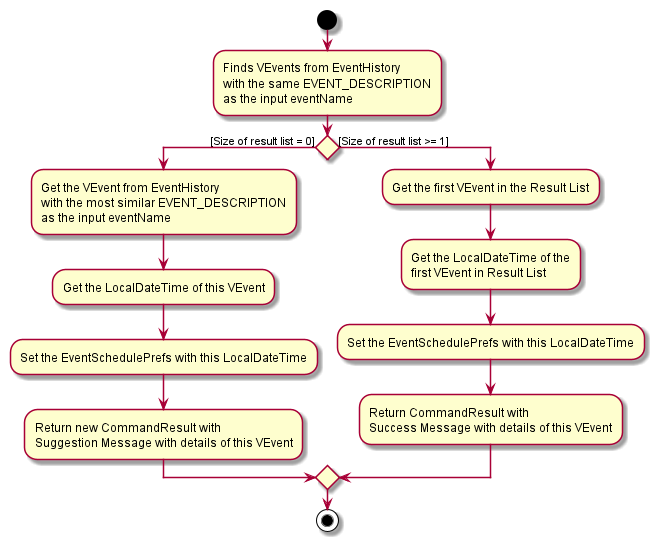
7.5.7. Schedule IndexAll Command
Implementation
The following is a detailed explanation of the operations which EventIndexAllCommand
performs.
Format for getting the indexes of all events is schedule indexAll .
|
Step 1: The EventIndexAllCommand#execute(Model model)
method is executed which obtains all indexes of the all of VEvents in the model.
This method first obtains the current list of Pair
of Index
and VEvents
in EventHistory#vEvents
using
Model#getAllVEventsWithIndex()
.
Step 2: The current list is then validated. If the list is empty, a new CommandResult
with message EventIndexAllCommand#MESSAGE_NO_EVENT
will be returned.
Step 3: The success message is generated using EventIndexAllCommand#generateResultMessage(List<Pair<Index, VEvent>> pairVEventIndexList)
and a new CommandResult
will be returned with this message.
The following Activity Diagram will provide a clearer picture on how the EventIndexAllCommand is supposed to run.
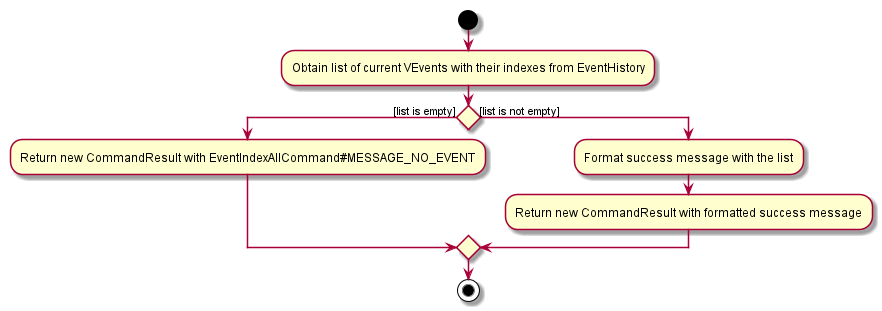
7.5.8. Schedule View Command
Implementation
The following is a detailed explanation of the operations which EventViewCommand
performs.
Format for changing the view mode of the schedule is schedule view mode/SCHEDULE_MODE date/YYYY-MM-DD .
|
SCHEDULE_MODE can only takes the values daily or weekly . Any other values will result in an error thrown.
|
Date must be entered in YYYY-MM-DD format. Any other values will result in an error thrown. |
Step 1: The EventViewCommand#execute(Model model)
method is executed which changes the view mode of the model.
This method sets EventSchedulePrefs#eventScheduleView
and EventSchedulePrefs#localDateTime
with EventViewCommand#scheduleViewMode
and EventViewCommand#targetViewDateTime
respectively.
Step 2: The success message is generated using EventViewCommand#generateSuccessMessage(EventScheduleView eventScheduleViewMode, LocalDateTime targetViewDate)
and a new CommandResult
will be returned with this message.
The following Activity Diagram will provide a clearer picture on how the EventViewCommand is supposed to run.
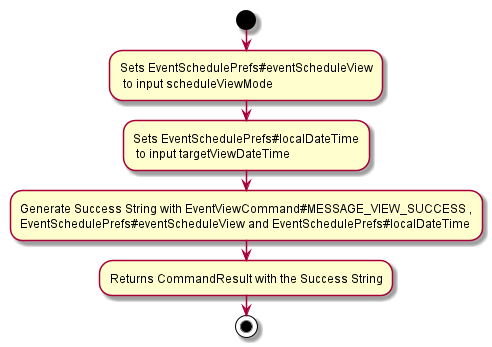
7.5.9. Schedule Export Command
Implementation
The following is a detailed explanation of the operations which EventExportCommand
performs.
Format for exporting the schedule into an .ics file is schedule export .
|
Step 1: The `The EventExportCommand#execute(Model model) method is executed which exports the events in the scheduler into a .ics file in the data folder.
Step 2: The command box will be reflected with the EventExportCommand#MESSAGE_SUCCESS constant and a new CommandResult will be returned with the message.
Step 3: Subsequently, the CommandResult will be processed by the`MainWindow` in the UI component and generate a mySchedule.ics file in the data folder of the current directory.
7.6. Logging
This section describes how TeaPet record it’s logs.
We are using java.util.logging
package for logging. The LogsCenter
class is used to manage the logging levels and logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file (See Section 7.7, “Configuration”) -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application -
WARNING
: Can continue, but with caution -
INFO
: Information showing the noteworthy actions by the App -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size
7.7. Configuration
Certain properties of the application can be controlled (e.g user prefs file location, logging level) through the configuration file (default: config.json
).
8. Documentation
Refer to the guide here.
9. Testing
Refer to the guide here.
10. Dev Ops
Refer to the guide here.
Appendix A: Product Scope
Target user profile:
-
form teacher of a class
-
has a need to manage a significant number of students
-
has a need to take the attendance of students
-
wants to be able to track the homework and progress of students
-
wants to be able to keep a schedule of his/her classes and events
-
wants to be able to keep track of students' behavior in class
-
prefer desktop applications over other types
-
can type fast
-
prefers typing over mouse input
-
is reasonably comfortable using CLI apps
Value proposition: Ability to manage students administration and personal commitments better than a typical mouse/GUI driven application. Overall increase in productivity.
Appendix B: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
|
new user |
see usage instructions |
refer to instructions when I forget how to use the App |
|
competent form teacher |
add students |
add new students to the class list |
|
form teacher |
delete a student |
remove students that I no longer need |
|
lazy form teacher |
find a student by name |
locate details of students without having to go through the entire list |
|
form teacher doing their job |
take the attendance of my students |
know who is present for my class |
|
organised form teacher |
have a schedule tracking my events |
know what I need to attend/do in a day |
|
diligent form teacher |
maintain of a list of students who have completed my homework |
know who has not submitted my homework |
|
caring form teacher |
take down notes for student’s behavior |
track the behaviour of my students |
|
hard working form teacher |
see the scores of my class |
track the academic progress of my class |
|
lazy form teacher |
sort students by alphabetical order |
locate a student easily |
|
form teacher |
update the details of my students |
make necessary changes to my student’s particulars |
|
well prepared form teacher |
maintain emergency contacts of my students |
know who to contact in case of emergency |
|
form teacher |
be able to view the available dates in chronological order that contains administrative information of the class |
know which are the available dates that I can view |
|
form teacher |
view the administrative information of the class on a specific date |
access the required administrative information |
|
form teacher |
be able to view my assessment list by the latest date |
know which are the more urgent or overdue assessments to monitor |
|
form teacher |
view the academic statistics of my class |
know the academic progress of my students |
|
form teacher |
set different colours to my events |
see clearer what type of events I have |
|
form teacher |
specify if a student is late or absent for class |
know why my student is absent |
|
form teacher |
hide private contact details by default |
minimize chance of someone else seeing them by accident |
|
caring form teacher |
export academic statistics into a printable file |
share and discuss the student’s academic progress with their parents |
|
caring form teacher |
export behavioural notes into a printable file |
share and discuss the student’s behaviour with their parents |
|
form teacher |
keep track of the sitting arrangement of the class |
students who change their seats unknowingly |
|
form teacher |
record the temperature of students |
track the health of my students |
|
form teacher |
get feedback from other teachers teaching the students of my class |
better understand the progress of the class |
Appendix C: Use Cases
(For all use cases below, the System is the TeaPet
and the Actor is the Teacher
, unless specified otherwise)
Use case: UC01 - Add student
MSS
-
User enters a student name, followed by optional attributes such as emergency contacts, through the command line.
-
TeaPet adds the student and his/her attributes to the class list.
-
TeaPet displays feedback to the user that a new student is being added.
Use case ends.
Extensions
-
1a. Student is invalid.
-
1a1. TeaPet shows an error message.
Use case ends.
-
-
1b. Student particulars keyed in by user are invalid.
-
1b1. TeaPet shows an error message.
Use case ends.
-
Use case: UC02 - Edit student
MSS
-
User specifies which student, using the name, and what particulars he/she wants to edit in the command line.
-
TeaPet edits the student’s particulars in the class list as instructed by the commands.
-
TeaPet displays feedback to the user that the student has been edited, followed by the changes made.
Use case ends.
Extensions
-
1a. Student is invalid.
-
1a1. TeaPet shows an error message.
Use case ends.
-
-
1b. Student particulars keyed in by user are invalid.
-
1b1. TeaPet shows an error message.
Use case ends.
-
Use case: UC03 - Delete student
MSS
-
User specifies which student, using the index, he/she wants to remove.
-
TeaPet removes the student from the class list.
-
TeaPet displays feedback to the user that the student is being removed.
Use case ends.
Extensions
-
1a. Student index entered by user is invalid.
-
1a1. TeaPet shows an error message.
Use case ends.
-
Use case: UC04 - Display Schedule
MSS
-
User keys in the command to display events.
-
TeaPet displays the events in chronological order.
Use case ends.
Extensions
-
1a. Command is invalid.
-
1a1. TeaPet shows an error message.
Use case ends.
-
Use case: UC05 - Display student class list.
MSS
-
User enters the command to display the class list.
-
TeaPet displays the class list with the students' tags, mobile number, email, and notes.
Use case ends.
Extensions
-
1a. Command is invalid.
-
1a1. TeaPet shows an error message.
Use case ends.
-
Use case: UC06 - Display admin class list.
MSS
-
User enters the command to display the administrative version of the class list.
-
TeaPet displays the class list with the students' attendance status and temperature.
Use case ends.
Extensions
-
1a. Command is invalid.
-
1a1. TeaPet shows an error message.
Use case ends.
-
Use case: UC07 - Deleting a date from the list of dates.
MSS
-
User enters the command to delete a specific date from the list of dates.
-
TeaPet searches through the list of dates for the date provided by the user.
-
TeaPet removes that date from the date list.
-
TeaPet displays that the date has been deleted successfully.
Use case ends.
Extensions
-
1a. Command is invalid.
-
1a1. TeaPet shows an error message.
Use case ends.
-
-
1b. Command is in incorrect format.
-
1b1. TeaPet shows an error message displaying the correct format for the command.
Use case ends.
-
-
1c. Date is in incorrect format.
-
1c1. TeaPet shows an error message displaying the correct format for date.
Use case ends.
-
-
2a. Date provided is not in the list of dates.
-
2a1. TeaPet shows an error message displaying date is not found.
Use case ends.
Use case: UC08 - Display detailed class list.
-
MSS
-
User enters the command to display the detailed version of the class list.
-
TeaPet displays the class list with all of the students' attributes.
Use case ends.
Extensions
-
1a. Command is invalid.
-
1a1. TeaPet shows an error message.
Use case ends.
-
Use case: UC09 - Display students' academic progress
MSS
-
User enters the command to display academic progress of students.
-
TeaPet displays the academic progress in chronological order.
Use case ends.
Extensions
-
1a. Command is invalid.
-
1a1. TeaPet shows an error message.
Use case ends.
-
Use case: UC10 - Add assessment to academics
MSS
-
User enters command, together with an assessment description, type and date.
-
TeaPet adds the new assessment and its details to the academics list.
-
TeaPet displays feedback to the user that a new assessment to the academics list.
Use case ends.
Extensions
-
1a. Assessment is invalid.
-
1a1. TeaPet shows an error message.
-
-
1b. Assessment details keyed by user are invalid.
-
1b1. TeaPet shows an error message.
Use case ends.
-
Use case: UC11 - Edit assessment
MSS
-
User specifies which assessment, using its index in the academics list, and what details he/she wants to edit in the command line.
-
TeaPet edits the assessment’s details in the academics list as instructed by the commands.
-
TeaPet displays feedback to the user that the assessment has been edited, followed by the changes made.
Use case ends.
Extensions
-
1a. Edited assessment is invalid.
-
1a1. TeaPet shows an error message.
Use case ends.
-
-
1b. Assessment details keyed in by user are invalid.
-
1b1. TeaPet shows an error message.
Use case ends.
-
Use case: UC12 - Delete assessment
MSS
-
User specifies which assessment, using the index, he/she wants to remove.
-
TeaPet removes the assessment from the class list.
-
TeaPet displays feedback to the user that the assessment is being removed.
Use case ends.
Extensions
-
1a. Assessment index entered by user is invalid.
-
1a1. TeaPet shows an error message.
Use case ends.
-
Use case: UC13 - Submit student’s work for assessment
MSS
-
User specifies which assessment and student, using index and name respectively, that he/she wants to submit work to.
-
TeaPet submits the student’s work to the assessment from the academics list.
-
TeaPet displays feedback to the user that the following student’s work has been submitted to the assessment.
Use case ends.
Extensions
-
1a. Assessment index entered by user is invalid.
-
1a1. TeaPet shows an error message.
Use case ends.
-
-
1b. Student name is invalid.
-
1b1. TeaPet shows an error message.
Use case ends.
-
-
1c. Student has already submitted their work.
-
1c1. TeaPet shows an error message.
Use case ends.
-
Use case: UC14 - Marks student’s work for assessment
MSS
-
User specifies which assessment and student, using index and name respectively, that he/she wants to mark.
-
TeaPet marks and stores the submission’s score to the assessment from the academics list.
-
TeaPet displays feedback to the user that the following student’s work has been marked and stored to the assessment.
Use case ends.
Extensions
-
1a. Assessment index entered by user is invalid.
-
1a1. TeaPet shows an error message.
Use case ends.
-
-
1b. Student name is invalid.
-
1b1. TeaPet shows an error message.
Use case ends.
-
-
1c. Student has yet to submit their work.
-
1c1. TeaPet shows an error message.
Use case ends.
-
Use case: UC15 - Add note for specific student
MSS
-
User enters command, together with a student name, note’s content and priority level.
-
TeaPet displays feedback that a new note is now tagged to the student specified.
-
TeaPet’s note panel will display the updated list of notes.
Use case ends.
Extensions
-
1a. Command is invalid.
-
1a1. TeaPet shows an error message.
-
-
1b. Student is invalid.
-
1b1. TeaPet shows an error message.
Use case ends.
-
Use case: UC16 - Edit note of specific student
MSS
-
User enters command, together with an index of the note to edit, and fields to update.
-
TeaPet displays feedback that the specific note chosen has been edited.
-
TeaPet’s note panel will display the updated list of notes.
Use case ends.
Extensions
-
1a. Command is invalid.
-
1a1. TeaPet shows an error message.
-
-
1b. Student is invalid.
-
1b1. TeaPet shows an error message.
-
-
1c. Index is invalid.
-
1c1. TeaPet shows an error message.
Use case ends.
-
Use case: UC17 - Delete note for specific student
MSS
-
User enters command, together with an index of the note to delete.
-
TeaPet displays feedback that the specifed note is deleted.
-
TeaPet’s note panel will display the updated list of notes.
Use case ends.
Extensions
-
1a. Command is invalid.
-
1a1. TeaPet shows an error message.
-
-
1b. Index is invalid.
-
1b1. TeaPet shows an error message.
Use case ends.
-
Use case: UC18 - Add event into schedule
MSS
-
Teacher wishes to add an event into the scheduler
-
Teacher enters the event details.
-
TeaPet saves the item and displays it on the scheduler.
Use case ends.
Extensions
-
2a. Item is missing details
-
2a1. Teapet displays an error message.
Use case resumes at step 2.
Use case ends.
-
Use case: UC19 - Delete event from schedule
Preconditions 1. Event exists in scheduler.
MSS
-
Teacher lists the events in calendar (UC12)
-
Teacher wishes to delete an event.
-
Teacher confirms the deletion.
-
TeaPet deletes the event from the scheduler.
Use case ends.
Extensions
-
2a. Teacher reconsiders and chooses not to remove the event.
Use case ends.
Use case: UC20 - Update student profile picture
Preconditions 1. Png files in image folder is in correct format.
MSS
-
Teacher wants to update image of students.
-
Teacher adds in the respective images into the image folder.
-
TeaPet confirms the process.
-
TeaPet updates the profile pictures of students in the student list.
Use case ends.
Appendix D: Non Functional Requirements
-
Should work on any mainstream OS as long as it has Java
11
or above installed. -
Should be able to hold up to 500 students without a noticeable sluggishness in performance for typical usage.
-
A teacher with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish majority of the tasks faster using commands than using the mouse.
-
TeaPet should be used only for a teacher handling his/her own form class, not by any other teachers.
-
TeaPet should be able to work without internet access.
-
The teacher should be able to familiarise himself/herself within half an hour of usage.
{More to be added}
Appendix E: Glossary
- Attributes
-
The information of a student. For example, phone number, address or next-of-kin contact details.
- Class List
-
Class list of students
- CLI
-
Command Line Interface
- GUI
-
Graphical User Interface
- Mainstream OS
-
Windows, Linux, Unix, OS-X
- Private contact detail
-
A contact detail that is not meant to be shared with others
- Schedule
-
TeaPet’s schedule that stores all of the teacher’s events
Appendix F: Product Survey
TeacherKit
Pros:
-
Functionality
-
Ease of data tracking
-
Tracks attendance and grades
-
-
Non-funtional requirements
-
Attractive looking GUI
-
Cross platform
-
Cons:
-
Functionality
-
Unable to tag notes to students
-
Unable to track behavioural score
-
Unable to show statistics on exam assessment
-
-
Non-functional requirements
-
Requires internet access
-
Some features are blocked by advertisements and pop ups
-
GUI-reliant, many buttons have to be pressed and many screens traversed to perform a task
-
Author: Simon Lam
Product information can be found at https://www.teacherkit.net/
Appendix G: Instructions for Manual Testing
Given below are instructions to test the app manually.
These instructions only provide a starting point for testers to work on; testers are expected to do more exploratory testing. |
G.1. Launch and Shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file
Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained. === Manual Test: Student particulars
-
-
Adding a student from class list with the specific name entered by user.
-
Test case:
student add name/John Tan Jun Wei phone/83391329 email/john@gmail.com temp/36.0 adr/Punggol Street 22 nok/James-father-93259589
Expected: Student John Tan Jun Wei has been added to the class list. -
Test case:
student add name/John Tan Jun Wei phone/83393129 email/john@gmail.com temp/3@.5 adr/Punggol Street 22 nok/James-father-93259589
Expected: No student is added. Error details shown in the status message. Status bar remains the same. -
Test case:
student add name/John Tan Jun Wei phone/839 email/john@gmail.com temp/36.0 adr/Punggol Street 22 nok/James-father-93259589
Expected: An error message is shown as the phone number of student is invalid. -
Test case:
student add name/John Tan Jun Wei phone/83391329 email/john@gmail.com temp/36.0 adr/Punggol Street 22 nok/James-dad-93259589
Expected: An error message is shown as the relationship of NOK is not recognised. -
Test case:
student add name/John333 phone/83391329 email/john@gmail.com temp/36.0 adr/Punggol Street 22 nok/James-father-93259589
Expected: An error message is shown as the name of student cannot contain numbers.
-
-
Deleting a student from class list with the specific name entered by user.
-
Test case:
student delete 1
Expected: The student at the first index is deleted from the list. Status message displays that the specified student has been deleted. -
Test case:
delete Tan John Wei Jun
Expected: No student is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
delete
,delete 10
(where the specified student is not a student in the class list due to the index being out of bounds.) {give more}
Expected: Similar to test case b.
-
G.2. Manual Test: Class Admin Particulars
-
Saving a date
-
Test case:
admin save
Expected: Date has either been saved if today’s date is not in the list of dates, or date not saved if today’s date is already in the list of dates. Status message either displays that today’s date has been saved or displays that current date already exists in the current list of dates respectively. -
Other incorrect delete commands to try:
save
,admin save DATE
whereDATE
is in YYYY-MM-DD format.
Expected: Status bar displays invalid command and incorrect command format message respectively.
-
-
Deleting a date
-
Test case:
admin delete DATE
where DATE is in YYYY-MM-DD format.
Expected: Date has either been deleted if the date provided exists in the list of dates, or no dates will be deleted if the date provided is not in the list. Status message either displays that the specific date has been deleted or displays that current date already exists in the current list of dates respectively. -
Other incorrect delete commands to try:
admin delete
,delete DATE
,admin delete DATE
, whereDATE
is in YYYY-MM-DD format andDATE
is in the wrongDATE
format.
Expected: Status bar displays invalid command and incorrect command format message.
-
G.3. Manual Test: Class Academics
-
Adding an assessment to academics
-
Prerequisites: The scheduler already contain an assessment with description "Math Differentiation Homework", type "homework", date "2020-03-23".
-
Test case:
academics add desc/Math Integration Homework type/homework date/2020-03-23
Expected: An assessment with the description Math Integration Homework is added to academics. -
Test case: `academics add desc/ `
Expected: An error message is shown due to invalid command. -
Test case:
academics add desc/Math Integration Homework type/ date/2020-03-23
Expected: An error message is shown due to invalid assessment type. -
Test case:
academics add desc/Math Integration Homework type/homework date/
Expected: An error message is shown due to invalid assessment date. -
Test case:
academics add desc/Math Integration Homework type/homework date/2020/03/04
Expected: An error message is shown due to invalid assessment date.
-
-
Editing an assessment in academics.
-
Test case:
academics edit 1 desc/Chinese Spelling Practice
Expected: First assessment in the academics list is updated with new description. Only the description should be modified. -
Test case:
academics edit 1 desc/Chinese Spelling Practice type/exam date/2020-03-04
Expected: First assessment in the academics list is updated with new description, type and date. -
Test case:
academics edit 1 desc/
Expected: An error message is shown due to invalid assessment description. -
Test case:
academics edit 1 desc/Chinese Spelling Practice type/assignment
Expected: An error message is shown due to invalid assessment type. -
Test case:
academics edit 1 date/2020/03/04
Expected: An error message is shown due to invalid assessment date.
-
-
Deleting an assessment
-
Test case:
academics delete INDEX
where INDEX is a valid index.
Expected: Assessment will be deleted if the given index is valid. The status message either displays that the specified assessment has been deleted or show that the index given is invalid. -
Other incorrect delete commands to try:
academics delete
Expected: Status bar displays invalid command and incorrect command format message.
-
-
Submitting a student’s work for an assessment
-
Test case:
academics submit INDEX stu/Simon_Lam
where INDEX is a valid index and Simon Lam is an existing student in TeaPet.
Expected: Simon Lam’s work will be submitted to the specified assessment if the given index is valid. The status message either displays that the student’s work has been submitted or show that the index given is invalid. -
Other incorrect submission commands to try:
academics submit
Expected: Status bar displays invalid command and incorrect command format message.
-
-
Marking a student’s work for an assessment
-
Test case:
academics mark INDEX stu/Simon_Lam-90
where INDEX is a valid index and Simon Lam is an existing student in TeaPet who scored 90 for the given assessment.
Expected: Simon Lam’s work will be marked and his score of 90 will be stored in the specified assessment if the given index is valid. The status message either displays that the student’s work has been marked or show that the index given is invalid. -
Other incorrect marking commands to try:
academics mark
Expected: Status bar displays invalid command and incorrect command format message.
-
-
Exporting academic statistics into .csv file.
-
Test case:
academics export
Expected: A file named studentAcademics.csv should be created in the data folder of the same directory in which TeaPet is installed.
-
G.4. Manual Test: Personal Schedule
-
Displaying the personal schedule
-
Test case:
schedule
Expected: User should now be able to view the schedule at the current date. A help message should also be shown in the command box.
-
-
Adding an event to the scheduler
-
Prerequisites: The scheduler already contain an event with name "Coffee Break", startDateTime "2020-04-04T12:00", endDateTime "2020-04-04T13:00". The recurrence type and color do not matter as long as they are valid.
-
Test case:
schedule add eventName/Consultation startDateTime/2020-04-10T10:00 endDateTime/2020-04-10T12:00 recur/none color/5
Expected: An event with name Consultation is added to the scheduler. -
Test case:
schedule add eventName/Coffee Break startDateTime/2020-04-04T12:00 endDateTime/2020-04-04T13:00 recur/none color/5
Expected: An error message is shown due to duplicate events being created. -
Test case: `schedule add eventName/ `
Expected: An error message is shown due to invalid command. -
Test case:
schedule add eventName/Consultation startDateTime/2020-04-10T10:00 endDateTime/2020-04-10T12:00 recur/none color/24
Expected: An error message is shown due to an invalid color code. -
Test case:
schedule add eventName/Consultation startDateTime/2020-04-10T10:00 endDateTime/2020-04-10T12:00 recur/fortnightly color/5
Expected: An error message is shown due to invalid recurrence type. -
Test case:
schedule add eventName/Consultation startDateTime/2020-04-10T13:00 endDateTime/2020-04-10T12:00 recur/none color/5
Expected: An error message is shown due to the invalid date time range.
-
-
Editing an event in the schedule
Prerequisite: The scheduler already contain an event with index 1, name "Coffee Break", startDateTime "2020-04-04T12:00", endDateTime "2020-04-04T13:00" . No other events exists. |
-
Test case:
schedule edit 1 eventName/Meeting at Town
Expected: The event name is changed to "Meeting at Town". The rest of the fields remains the same as before the edit command was called. -
Test case:
schedule edit 1 endDateTime/2020-04-04T14:00
Expected: The event end date time is extended to 1400 hrs. The rest of the fields remains the same as before the edit command was called. -
Test case:
schedule edit 2 eventName/Meeting now
Expected: An error message due to invalid index provided. -
Test case:
schedule edit 2 eventName/
Expected: An error message due to invalid event name entered. -
Test case:
schedule edit 1
Expected: An error message due to no fields provided to edit.-
Deleting an event in the schedule
-
Prerequisite: The scheduler already contain an event with index 1, name "Coffee Break", startDateTime "2020-04-04T12:00", endDateTime "2020-04-04T13:00" . No other events exists. |
-
Test case:
schedule delete 1
Expected: The event with name "Coffee Break" is removed from the schedule. -
Test case:
schedule delete 2
Expected: An error message due to invalid index provided. -
Test case:
schedule delete a
Expected: An error message due to wrong command format provided. -
Test case:
schedule delete
Expected: An error message due to wrong command format provided.-
Getting the index of an event in the schedule
-
Prerequisite: The scheduler already contain an event with index 1, name "Coffee Break", startDateTime "2020-04-04T12:00", endDateTime "2020-04-04T13:00" . No other events exists. |
-
Test case:
schedule indexGet/Coffee Break
Expected: The event description along with its index is shown in the command box. -
Test case:
schedule indexGet/coffee
Expected: The event description with the closest name to the search term "coffee" is displayed along with its index in the command box. -
Test case:
schedule indexGet/i am not trying to find
Expected: By default, the event with index 1 is displayed. Since no events match the search term. -
Test case:
schedule indexGet/
Expected: An error message due to invalid event name provided.-
Getting the indexes of all events
-
Prerequisite: The scheduler already contain an event with index 1, name "Coffee Break", startDateTime "2020-04-04T12:00", endDateTime "2020-04-04T13:00" and another event with index 2, name "Dinner", startDateTime "2020-04-04T17:00", endDateTime "2020-04-04T18:00" |
-
Test case:
schedule indexAll
Expected: Both event’s details along with their indexes will be shown in the command box.-
Changing the view mode of the schedule
-
-
Test case:
schedule view mode/weekly date/2020-04-20
Expected: The schedule will show the week of Monday to Sunday that reference date "2020-04-20" lies on. -
Test case:
schedule view mode/daily date/2020-04-21
Expected: The schedule will show all the events on the reference date "2002-04-21". -
Test case:
schedule view mode/daily
Expected: An error message due to wrong command format provided. -
Test case:
schedule view date/2020-04-21
Expected: An error message due to wrong command format provided. -
Test case:
schedule view
Expected: An error message due to wrong command format provided.-
Exporting the schedule into an .ics file
-
-
Test case
schedule export
Expected: An .ics file with name "mySchedule.ics" will be created in the data folder in the current directory of TeaPet.
G.5. Manual Test: Notes
In order to add or edit a specific note belonging to a student, the student must first be in the class-list. In order for optimal manual testing, please create the student first before creating a note which belongs to him/her. You can refer to Section 7.1.1, “Student Add command” to find out more on how to add a student to the class-list. |
Delete all previous notes. Be sure two students named Freddy Zhang and Lee Hui Ting are in the class-list. Conduct these tests sequentially from first to last, for the most effective testing experience. |
-
Adding a note to the notes panel.
-
Test case:
notes add name/Freddy Zhang cont/Reminder to print his testimonial pr/HIGH
Expected: New note added to the notes panel. This note should be red (high priority) in colour, belonging to Freddy Zhang. The timestamp in the note should be the current date and time. -
Test case:
notes add name/Freddy Zhang cont/Freddy was late for class for the second day in a row. pr/LOW
Expected: New note added to the notes panel. This note should be yellow (low priority) in colour, belonging to Freddy Zhang. The timestamp in the note should be the current date and time. -
Test case:
notes add name/Lee Hui Ting cont/Hui Ting left school for an interview. pr/MEDIUM
Expected: New note added to the notes panel. This note should be orange (medium priority) in colour, belonging to Lee Hui Ting. The timestamp in the note should be the current date and time. -
Test case:
notes add name/Freddy_Zhang_ cont/He exemplified an improvement in behaviour pr/LOW
Expected: An error message is shown as the student name should be alpha-numeric. -
Test case:
notes add name/Freddy Zhang cont/He exemplified an improvement in behaviour pr/Not High
Expected: An error message is shown as priority indicated must be either LOW, MEDIUM or HIGH, case-insensitive.
-
-
Editing a note in the notes panel.
-
Test case:
notes edit 1 cont/Reminder to print his testimonial and gradebook
Expected: First note in the panel is updated with new content. Only the content should be modified, and nothing else. -
Test case:
notes edit 1 name/Lee Hui Ting cont/Reminder to print her testimonial and gradebook
Expected: First note in the panel is updated with new name and content. Note should reflect student Lee Hui Ting’s name, with modfied content. Nothing else should be different. -
Test case:
notes edit 1 pr/MEDIUM
Expected: First note in the panel is updated new priority. Note colour should change from red to orange.
-
-
Deleting a note in the notes panel.
-
Test case:
notes delete 1
Expected: First note in the panel should be removed. -
Test case:
notes delete 0
Expected: An error message is shown as the integer provided must be greater than zero. -
Test case:
notes delete 4
Expected: An error message is shown as the integer provided as the number of notes in the notes panel is less than 4. Integer provided is out of range.
-
-
Filtering the list of notes to search for specific notes.
-
Test case:
notes filter Freddy
Expected: Only notes containing the word Freddy should be displayed in the notes panel. -
Test case:
notes filter low
Expected: Only notes containing the word low should be displayed in the notes panel.
-
-
Exporting notes in the notes panel into a .csv file.
-
Test case:
notes export
Expected: A file named studentNotes.csv should be created in the data folder of the same directory in which TeaPet is installed. -
Test case:
notes export 3
Expected: An error message is shown as there should be no arguments passed into notes export command.
-
Appendix H: Effort
Overview
Our team wanted to make an application to create a personal assistant to assist form teachers in their highly
stressful profession. We decided to build
TeaPet to easily manage students and their schedule both inside and outside of classrooms. TeaPet is
significantly different from Address Book 3 (AB3). In addition to the existing basic features of AB3, we had to implement
additional features to satisfy the needs of our target audience, form teachers. Features that are unique to TeaPet are
student particulars, student administration, academics tracking, notes keeping, and a easy to use personal scheduler.
Challenges faced
-
Building TeaPet upon AB3 - Initially, our group took a long time understanding the logic of AB3. In addition, converting our own ideas into code and building upon it on AB3 was very tough. We took a long time familiarising with the the flow of the code, hence resulting in a slow development of TeaPet initially.
-
Debugging - Each of us was doing our own individual features, hence when we were merging our pull requests, there are often merge conflicts. Furthermore, as there are are some inter-dependencies between our features, when one person changes his or her code, the other party gets affected. Also, when we were doing quality assurance, we spent a long time figuring out why some of the test cases fail. However, that helped us better understand our code and its expected behaviour, which proved to be a big help when building TeaPet
-
Documentation - Initially, it was a challenge to standardise all our format and images in both the user guide and the developer guide due to different documentation styles and formats. To tackle this issue, we often skyped for extended periods of time to go through the documents in detail. More often than not, one of us would share their screen and we would break down the document line by line. After much hardwork, we were finally able to standardise and complete our documentation
Achievements
-
Integration of 5 unique features - The team had set out with the plan to develop TeaPet with 5 unique, user-centric features. They are namely student particulars, student administration, student academics, notes and personal scheduler. Throughout the project, we made consistent effort to ensure every feature worked well with one another, in seamless fashion. There were certain scenarios where the codebase broke due to associations and dependencies. Despite that, the team manage to ensure all 5 features were well integrated in the final version of TeaPet, seeking to provide a fantastic user experience.
-
Maintaining test coverage – The team had decided to not only ensure our application is well-functioning, but also resistant to bugs. We applied the testing methods taught in the module to write test files for our classes. It is an achievement as despite our large code base, we had managed to achieve our target of 70% test coverage, extensively covering the features.
Conclusion
In conclusion, our team spent great amounts of effort and we are proud to produce TeaPet by working together.
Overall, we believe that TeaPet is a good testament of our hard work and time spent to perfect it.